单层感知机的设计与实现python
时间: 2023-07-29 19:07:05 浏览: 134
单层感知机是一种最简单的神经网络,它只有一个神经元。在这里我将演示如何用Python实现单层感知机。
首先,我们需要引入一些库:
```python
import numpy as np
```
然后,我们定义一个Perceptron类,其中包含三个方法:`__init__`,`fit`和`predict`。`__init__`方法用于初始化模型参数,`fit`方法用于训练模型,`predict`方法用于预测结果。
```python
class Perceptron(object):
def __init__(self, learning_rate=0.1, max_iter=200):
self.learning_rate = learning_rate
self.max_iter = max_iter
def fit(self, X, y):
n_samples, n_features = X.shape
self.weights = np.zeros(n_features)
self.bias = 0
for _ in range(self.max_iter):
for idx, x_i in enumerate(X):
linear_output = np.dot(x_i, self.weights) + self.bias
if linear_output > 0:
y_pred = 1
else:
y_pred = 0
update = self.learning_rate * (y[idx] - y_pred)
self.weights += update * x_i
self.bias += update
def predict(self, X):
linear_output = np.dot(X, self.weights) + self.bias
y_pred = np.where(linear_output > 0, 1, 0)
return y_pred
```
以上就是单层感知机的模型代码,接下来是如何使用这个模型进行分类。首先,我们需要准备一些数据:
```python
X = np.array([[0,0], [0,1], [1,0], [1,1]])
y = np.array([0, 0, 0, 1])
```
上述代码中,`X`是输入数据,`y`是标签。
然后,我们可以创建一个Perceptron对象,并使用`fit`方法对模型进行训练:
```python
perceptron = Perceptron()
perceptron.fit(X, y)
```
最后,我们可以使用`predict`方法对新的数据进行分类:
```python
X_new = np.array([[0,0], [0,1], [1,0], [1,1]])
print(perceptron.predict(X_new))
```
以上代码输出的结果是`[0 0 0 1]`,表示模型成功地对输入数据进行了分类。
相关推荐
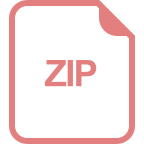
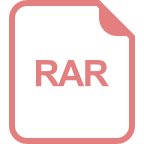














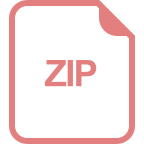