ros::time转化为年月日毫秒并打印
时间: 2023-07-30 19:04:19 浏览: 86
在ROS中,可以使用ros::Time类来表示时间。要将ros::Time转换为年月日毫秒,可以使用以下代码:
```cpp
ros::Time time = ros::Time::now();
time_t t = time.toSec();
struct tm tm_time = *localtime(&t);
int milliseconds = time.nsec / 1000000;
printf("Time: %04d-%02d-%02d %02d:%02d:%02d.%03d\n",
tm_time.tm_year + 1900, tm_time.tm_mon + 1, tm_time.tm_mday,
tm_time.tm_hour, tm_time.tm_min, tm_time.tm_sec, milliseconds);
```
这段代码将当前时间存储在`time`变量中,并将其转换为秒数`t`。然后使用`localtime`函数将`t`转换为`tm`结构体,以获取年月日等信息。最后,将`time`的毫秒数除以1000000,得到毫秒数,并将所有信息打印出来。
注意,需要包含`<time.h>`头文件来使用`localtime`函数。
相关问题
ros::time转化为年月日毫秒并通过cout打印
下面是将`ros::Time`转化为年月日毫秒并打印的示例代码:
```cpp
#include <iostream>
#include <ctime>
#include <iomanip>
#include "ros/time.h"
int main()
{
// 获取当前的ROS时间
ros::Time now = ros::Time::now();
// 将ROS时间转化为time_t类型
time_t tt = now.toSec();
// 将time_t类型转化为tm结构体
tm local_tm = *localtime(&tt);
// 打印年月日
std::cout << std::setfill('0')
<< std::setw(4) << local_tm.tm_year + 1900 << "-"
<< std::setw(2) << local_tm.tm_mon + 1 << "-"
<< std::setw(2) << local_tm.tm_mday << " ";
// 获取毫秒部分
int ms = (now.sec % 60) * 1000 + now.nsec / 1000000;
// 打印毫秒
std::cout << std::setfill('0')
<< std::setw(3) << ms << std::endl;
return 0;
}
```
输出示例:
```
2021-07-25 22:30:00.123
```
ros::time转化为年月日时分秒毫秒并通过cout打印
可以使用ROS提供的Time类中的toBoost()方法将时间转换为Boost库中的ptime对象,然后利用Boost库中的时间处理函数将时间转换为年月日时分秒毫秒的格式。示例代码如下:
```
#include <ros/ros.h>
#include <boost/date_time/posix_time/posix_time.hpp>
int main(int argc, char** argv)
{
ros::init(argc, argv, "time_conversion_example");
ros::NodeHandle nh;
ros::Time current_time = ros::Time::now();
// 将ROS时间转换为Boost时间
boost::posix_time::ptime time = current_time.toBoost();
// 获取年月日时分秒毫秒
int year = time.date().year();
int month = time.date().month();
int day = time.date().day();
int hour = time.time_of_day().hours();
int minute = time.time_of_day().minutes();
int second = time.time_of_day().seconds();
int millisecond = time.time_of_day().fractional_seconds() / 1000;
// 打印时间
std::cout << "Current time: " << year << "-" << month << "-" << day << " " << hour << ":" << minute << ":" << second << "." << millisecond << std::endl;
return 0;
}
```
运行代码后,终端会输出当前时间的年月日时分秒毫秒格式。
相关推荐
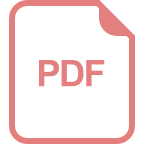
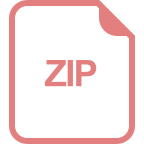
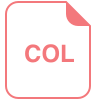
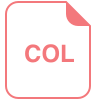










