搭建一个卷积神经网络的代码
时间: 2023-09-16 19:14:29 浏览: 92
以下是使用TensorFlow搭建一个简单的卷积神经网络的代码:
```python
import tensorflow as tf
# 加载数据集
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
# 数据预处理
x_train = x_train.reshape(x_train.shape[0], 28, 28, 1).astype('float32') / 255
x_test = x_test.reshape(x_test.shape[0], 28, 28, 1).astype('float32') / 255
y_train = tf.keras.utils.to_categorical(y_train, 10)
y_test = tf.keras.utils.to_categorical(y_test, 10)
# 定义卷积神经网络结构
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Conv2D(64, (3, 3), activation='relu'),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# 设置超参数
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
# 训练模型
model.fit(x_train, y_train, epochs=5, validation_data=(x_test, y_test))
# 评估模型
test_loss, test_acc = model.evaluate(x_test, y_test)
print('Test accuracy:', test_acc)
# 使用模型进行预测
predictions = model.predict(x_test[:5])
print(predictions)
```
在这个代码中,我们使用MNIST数据集搭建了一个简单的卷积神经网络,包含3个卷积层和2个全连接层。我们使用了TensorFlow提供的高层次API,如tf.keras.Sequential()和tf.keras.layers.Conv2D()等,简化了网络结构的定义过程。在训练和评估模型时,我们使用了交叉熵损失函数和准确率指标。最后,我们使用训练好的模型对测试集进行了预测,并输出了预测结果。
阅读全文
相关推荐
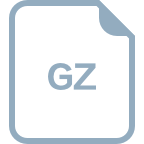




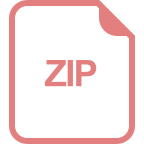
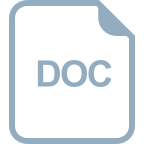








