#include <iostream> #include<string> using namespace std; struct stuinfo { int id; // 学号 string name; // 姓名 char gender; // 性别 int score1; // 课程1成绩 int score2; // 课程2成绩 int score3; // 课程3成绩 int score4; // 课程4成绩 }; double statistics(stuinfo stus[], int n) { } int main() { int n; stuinfo ptr[11] = { {104, "Zhang San", 'f', 94, 97, 91, 94}, {105, "Li Si", 'm', 90, 86, 90, 75}, {106, "Wang Wu", 'm', 94, 88, 91, 86}, {107, "Zhzo Liu", 'f', 92, 95, 81, 87}, {108, "Qian, Jiu", 'f', 99, 90, 82, 91}, {109, "Ding Yi", 'm', 98, 86, 80, 94}, {110, "Zhou Er", 'f', 90, 90, 91, 93}, {111, "Chen Ba", 'm', 92, 83, 92, 77}, {112, "Zhu Qi", 'f', 90, 85, 88, 81}, {113, "Wu Shi", 'm', 91, 82, 89, 86}, {114, "Du Du", 'm', 93, 84, 82, 87} }; cin >> n; cout << statistics(ptr, n) << endl; return 0; }在此基础上实现某班共有若干名学生,学生信息用如下的stuinfo结构表示, 其中,男学生则gender取值为’m’,女学生则gender取值为’f’。 2022年春季学期4门课程的成绩依次保存在score1~score4中。 请编写函数double statistics(stuinfo stus[], int n) 分别统计男生4门课程总分的平均分,以及女生4门课程总分的平均分, 其中stus数组存储了班上所有学生的信息,n为班上学生的总人数。 函数最后返回男生总分平均分减去女生总分平均分的结果(浮点数)。
时间: 2023-09-23 17:04:22 浏览: 69
下面是按要求实现函数`statistics()`的代码:
```cpp
#include <iostream>
#include <string>
using namespace std;
struct stuinfo {
int id; // 学号
string name; // 姓名
char gender; // 性别
int score1; // 课程1成绩
int score2; // 课程2成绩
int score3; // 课程3成绩
int score4; // 课程4成绩
};
double statistics(stuinfo stus[], int n)
{
double maleTotal = 0; // 男生总分
double femaleTotal = 0; // 女生总分
int maleCount = 0; // 男生人数
int femaleCount = 0; // 女生人数
// 遍历学生数组,统计男生总分和人数,女生总分和人数
for (int i = 0; i < n; i++) {
if (stus[i].gender == 'm') {
maleTotal += stus[i].score1 + stus[i].score2 + stus[i].score3 + stus[i].score4;
maleCount++;
} else if (stus[i].gender == 'f') {
femaleTotal += stus[i].score1 + stus[i].score2 + stus[i].score3 + stus[i].score4;
femaleCount++;
}
}
double maleAverage = maleTotal / (maleCount * 4); // 男生平均分
double femaleAverage = femaleTotal / (femaleCount * 4); // 女生平均分
return maleAverage - femaleAverage;
}
int main()
{
int n;
stuinfo ptr[11] = {
{104, "Zhang San", 'f', 94, 97, 91, 94},
{105, "Li Si", 'm', 90, 86, 90, 75},
{106, "Wang Wu", 'm', 94, 88, 91, 86},
{107, "Zhzo Liu", 'f', 92, 95, 81, 87},
{108, "Qian, Jiu", 'f', 99, 90, 82, 91},
{109, "Ding Yi", 'm', 98, 86, 80, 94},
{110, "Zhou Er", 'f', 90, 90, 91, 93},
{111, "Chen Ba", 'm', 92, 83, 92, 77},
{112, "Zhu Qi", 'f', 90, 85, 88, 81},
{113, "Wu Shi", 'm', 91, 82, 89, 86},
{114, "Du Du", 'm', 93, 84, 82, 87}
};
cin >> n;
cout << statistics(ptr, n) << endl;
return 0;
}
```
在上述代码中,函数`statistics()`统计男生4门课程总分的平均分和女生4门课程总分的平均分,并返回男生总分平均分减去女生总分平均分的结果。函数遍历学生数组,根据学生的性别将成绩累加到对应的总分中,并统计男生和女生的人数。然后,计算男生和女生的平均分。最后,返回男生总分平均分减去女生总分平均分的结果。
注意:在计算平均分时,需要将每个学生的4门课程成绩累加起来,并除以对应的人数得到平均分。
阅读全文
相关推荐
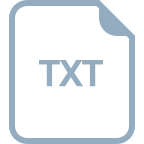
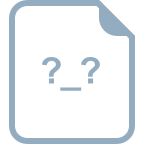
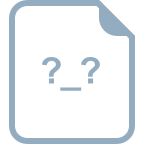
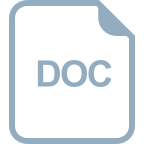
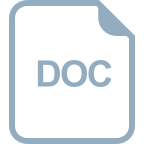
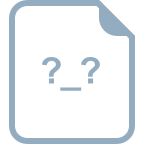
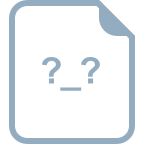
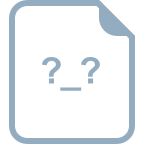
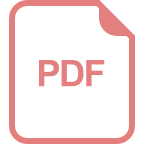
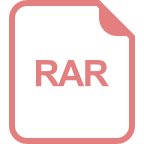
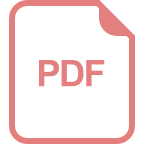
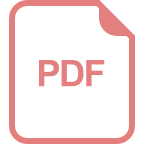
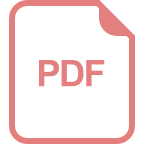
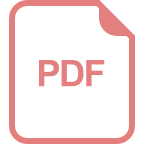
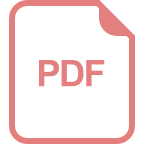
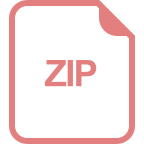
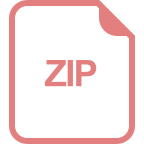
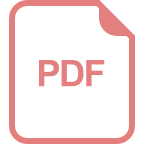