编写程序定义Point类,在类中定义整型的私有成员变量_x,_y,并定义成员函数Point&.operator++();和Point operator++(int),以实现对Point类重载“++”(自增)运ay( ) 算符,实现对坐标值的改变。 如果希望将“十十”(自增)运算符重载为友元函数,如何实现?
时间: 2023-06-12 16:07:59 浏览: 88
下面是定义Point类并重载自增运算符的示例代码:
```c++
#include<iostream>
using namespace std;
class Point{
private:
int _x;
int _y;
public:
Point(int x=0, int y=0):_x(x), _y(y){}
Point& operator++(){ // 前置自增运算符重载
_x++;
_y++;
return *this;
}
Point operator++(int){ // 后置自增运算符重载
Point tmp(*this);
_x++;
_y++;
return tmp;
}
friend Point& operator--(Point& p); // 友元函数前置自减运算符重载
friend Point operator--(Point& p, int); // 友元函数后置自减运算符重载
void print(){
cout << "(" << _x << ", " << _y << ")" << endl;
}
};
Point& operator--(Point& p){ // 友元函数前置自减运算符重载
p._x--;
p._y--;
return p;
}
Point operator--(Point& p, int){ // 友元函数后置自减运算符重载
Point tmp(p);
p._x--;
p._y--;
return tmp;
}
int main(){
Point p(1, 2);
cout << "p: ";
p.print(); // (1, 2)
++p;
cout << "++p: ";
p.print(); // (2, 3)
p++;
cout << "p++: ";
p.print(); // (3, 4)
--p;
cout << "--p: ";
p.print(); // (2, 3)
p--;
cout << "p--: ";
p.print(); // (1, 2)
return 0;
}
```
如果希望将自增运算符重载为友元函数,则需要将重载函数定义在类外,并在函数声明前加上关键字`friend`,示例如下:
```c++
class Point{
private:
int _x;
int _y;
public:
Point(int x=0, int y=0):_x(x), _y(y){}
friend Point& operator++(Point& p); // 友元函数前置自增运算符重载
friend Point operator++(Point& p, int); // 友元函数后置自增运算符重载
};
Point& operator++(Point& p){ // 友元函数前置自增运算符重载
p._x++;
p._y++;
return p;
}
Point operator++(Point& p, int){ // 友元函数后置自增运算符重载
Point tmp(p);
p._x++;
p._y++;
return tmp;
}
```
相关推荐
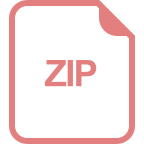
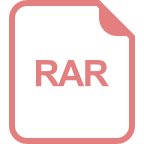
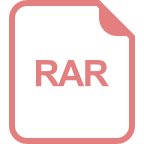





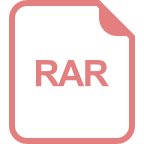
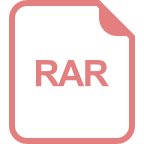
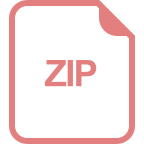
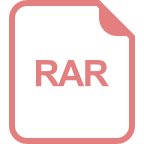
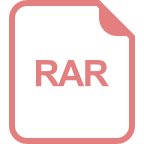
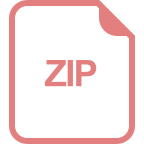
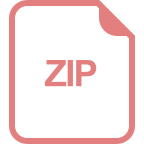