在上面的代码上添加参数设置,参数包括时钟频率、波特率、校验位、停止位
时间: 2023-07-10 13:24:36 浏览: 46
在上面的代码基础上,可以添加一些参数来灵活地配置 UART 的参数。下面是一个带有参数设置的 UART 驱动的示例代码:
```
module uart (
input clk,
input rst,
input rx,
output tx,
parameter CLK_FREQ = 50000000, // 时钟频率
parameter BAUD_RATE = 115200, // 波特率
parameter PARITY = 0, // 校验位 (0: 无校验, 1: 奇校验, 2: 偶校验)
parameter STOP_BITS = 1 // 停止位 (1: 1位停止位, 2: 2位停止位)
);
reg [7:0] data;
reg [2:0] state;
reg [3:0] count;
reg tx_busy;
parameter IDLE = 0, START = 1, DATA = 2, STOP = 3;
// 计算波特率分频值
localparam BAUD_DIV = CLK_FREQ / (BAUD_RATE * 16);
always @(posedge clk) begin
if (rst) begin
state <= IDLE;
count <= 0;
tx_busy <= 0;
tx <= 1;
end else begin
case (state)
IDLE: begin
if (!rx) begin
state <= START;
count <= 0;
end else if (tx_busy) begin
tx <= 0;
end else begin
tx <= 1;
end
end
START: begin
if (count < 8) begin
data[count] <= rx;
count <= count + 1;
end else begin
state <= DATA;
count <= 0;
end
end
DATA: begin
if (count < 8) begin
count <= count + 1;
end else begin
state <= STOP;
count <= 0;
end
end
STOP: begin
if (count < STOP_BITS) begin
count <= count + 1;
end else begin
state <= IDLE;
count <= 0;
tx_busy <= 0;
end
end
endcase
end
end
always @(posedge clk) begin
if (rst) begin
data <= 0;
end else begin
case (state)
IDLE: begin
if (tx_busy) begin
tx <= 0;
end
end
START: begin
tx <= 0;
end
DATA: begin
tx <= data[count];
end
STOP: begin
tx <= 1;
end
endcase
end
end
always @(posedge clk) begin
if (rst) begin
tx_busy <= 0;
end else begin
case (state)
START: begin
tx_busy <= 1;
end
STOP: begin
tx_busy <= 0;
end
endcase
end
end
endmodule
```
在这个代码中,我们添加了四个参数:时钟频率、波特率、校验位和停止位。波特率参数用来计算波特率分频值,校验位和停止位参数用来控制 UART 输入和输出的数据格式。代码中使用了 `localparam` 来定义了一个局部参数 `BAUD_DIV`,它表示了波特率分频值。在接下来的代码中,我们使用这个参数来控制 UART 的数据传输速率。
相关推荐
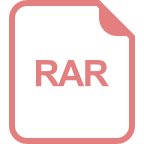
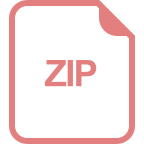
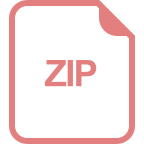
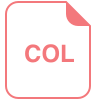
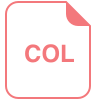
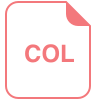












