#include "stdio.h" #include "stdlib.h" typedef int keytype; typedef struct node { keytype data; struct node *lchild, *rchild; }BitTree; //查找 int search(BitTree *T,keytype x,BitTree **F,BitTree **C) { *F=*C=NULL; while(T) { if(T->data==x) { *C=T; return 1;} *F=T; if(x<T->data) T=T->lchild; else T=T->rchild; } return 0; } //插入 int insert(BitTree **T,keytype x) { BitTree *F,*C,*s; if(search(*T,x,&F,&C)) return 0; s=(BitTree *)malloc(sizeof(BitTree)); s->data=x; s->lchild=s->rchild=NULL; if(F==NULL) { *T=s; return 1;} if(x<F->data) F->lchild=s; else F->rchild=s; return 1; } //建立 BitTree *creBST(void) { keytype x; BitTree *T=NULL; printf("Input datas(-1:End):"); scanf("%d",&x); while(x!=-1) { insert(&T,x); scanf("%d",&x); } return T; } //中序 void inorder(BitTree *T) { if(T) { inorder(T->lchild); printf("%5d",T->data); inorder(T->rchild); } } //删除 int delx(BitTree **T,keytype x) { BitTree *F,*p,*q,*s; if(!search(*T,x,&F,&p)) return 0; if(p->lchild==NULL) { q=p; p=p->rchild;} else if(p->rchild==NULL) { q=p; p=p->lchild;} else { q=p; s=q->lchild; while(s->rchild) { q=s; s=s->rchild;} p->data=s->data; if(q==p) q->lchild=s->lchild; else q->rchild=s->lchild; q=s; } if(F==NULL) *T=p; else if(q!=s) if(x<F->data) F->lchild=p; else F->rchild=p; free(q); return 1; }生成主函数
时间: 2024-03-13 10:44:33 浏览: 119
以下是一个使用上述代码的主函数示例:
```c
#include "stdio.h"
#include "stdlib.h"
typedef int keytype;
typedef struct node {
keytype data;
struct node *lchild, *rchild;
} BitTree;
// 查找
int search(BitTree *T, keytype x, BitTree **F, BitTree **C) {
*F = *C = NULL;
while (T) {
if (T->data == x) {
*C = T;
return 1;
}
*F = T;
if (x < T->data)
T = T->lchild;
else
T = T->rchild;
}
return 0;
}
// 插入
int insert(BitTree **T, keytype x) {
BitTree *F, *C, *s;
if (search(*T, x, &F, &C))
return 0;
s = (BitTree *)malloc(sizeof(BitTree));
s->data = x;
s->lchild = s->rchild = NULL;
if (F == NULL) {
*T = s;
return 1;
}
if (x < F->data)
F->lchild = s;
else
F->rchild = s;
return 1;
}
// 建立
BitTree *creBST(void) {
keytype x;
BitTree *T = NULL;
printf("Input datas(-1:End):");
scanf("%d", &x);
while (x != -1) {
insert(&T, x);
scanf("%d", &x);
}
return T;
}
// 中序遍历
void inorder(BitTree *T) {
if (T) {
inorder(T->lchild);
printf("%5d", T->data);
inorder(T->rchild);
}
}
// 删除
int delx(BitTree **T, keytype x) {
BitTree *F, *p, *q, *s;
if (!search(*T, x, &F, &p))
return 0;
if (p->lchild == NULL) {
q = p;
p = p->rchild;
} else if (p->rchild == NULL) {
q = p;
p = p->lchild;
} else {
q = p;
s = q->lchild;
while (s->rchild) {
q = s;
s = s->rchild;
}
p->data = s->data;
if (q == p)
q->lchild = s->lchild;
else
q->rchild = s->lchild;
q = s;
}
if (F == NULL)
*T = p;
else if (q != s)
if (x < F->data)
F->lchild = p;
else
F->rchild = p;
free(q);
return 1;
}
// 主函数
int main(void) {
BitTree *T = creBST(); // 建立二叉查找树
printf("Inorder: ");
inorder(T); // 中序遍历
printf("\n");
int del_num;
printf("Input the number you want to delete: ");
scanf("%d", &del_num);
if (delx(&T, del_num))
printf("Delete %d success.\n", del_num);
else
printf("Delete %d failed.\n", del_num);
printf("Inorder: ");
inorder(T); // 中序遍历
printf("\n");
return 0;
}
```
在这个主函数中,我们首先调用 `creBST` 函数建立二叉查找树,然后使用 `inorder` 函数中序遍历并打印出所有节点的值。接着,我们从用户输入中读取一个数字,调用 `delx` 函数从二叉查找树中删除该数字。最后再次使用 `inorder` 函数中序遍历并打印出所有节点的值,以验证删除操作是否成功。
阅读全文
相关推荐






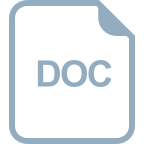










