在Dev c++中应用单链表设计一个100位以内的长整数加减运算的程序,输入输出数据每四位一组,组间用逗号分隔,加和减分别用不同的函数实现,程序应考虑输入数据的符号,请写出完整代码
时间: 2024-09-07 14:02:52 浏览: 63
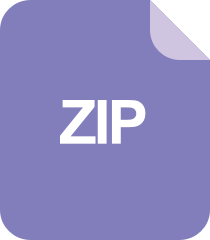
线性表+应用+多项式+dev c++
在Dev C++中,我们可以使用单链表来存储大整数,因为链表可以动态地处理任意长度的数据。以下是一个简单的示例,展示了如何创建一个类`BigInt`来表示和操作链表形式的长整数,以及实现加法和减法功能:
```cpp
#include <iostream>
#include <sstream>
// 定义节点结构
struct ListNode {
int digit;
ListNode* next;
};
class BigInt {
private:
ListNode* head;
bool isNegative;
public:
BigInt() : head(nullptr), isNegative(false) {}
// 添加数字到链表的末尾
void addDigit(int d) {
if (!head)
head = new ListNode{d, nullptr};
else {
ListNode* temp = head;
while (temp->next) {
temp = temp->next;
}
temp->next = new ListNode{d, nullptr};
}
}
// 转换链表为字符串,用于输出
std::string toStr() {
std::ostringstream oss;
ListNode* temp = head;
while (temp) {
oss << temp->digit;
temp = temp->next;
if (temp)
oss << ",";
}
return oss.str();
}
// 合并两个链表
static BigInt merge(BigInt a, BigInt b) {
if (a.isNegative != b.isNegative) {
a.isNegative = !a.isNegative; // 根据负数规则调整一个
} else {
if (a.head && b.head && a.head->digit > b.head->digit) { // 如果b较大,则交换
BigInt temp = a;
a = b;
b = temp;
}
}
ListNode* tail = a.head;
while (a.head && b.head) {
a.addDigit(a.head->digit + b.head->digit);
a.head = a.head->next;
b.head = b.head->next;
}
if (b.head)
a.addDigit(b.head->digit);
return a;
}
// 加法
BigInt add(const BigInt& other) {
return merge(*this, other);
}
// 减法
BigInt subtract(const BigInt& other) {
if (isNegative != other.isNegative) {
other.isNegative = !other.isNegative; // 确保其他是负数
} else {
other = -other; // 如果都是正数,取绝对值相减
}
return add(other);
}
// 输出链表
friend std::ostream& operator<<(std::ostream& os, const BigInt& bi) {
os << bi.toStr();
return os;
}
};
// 主函数示例
int main() {
BigInt num1, num2;
char input[5];
int sign = 1;
std::cout << "请输入第一个100位以内长整数,每四位一组,以逗号分隔: ";
std::getline(std::cin, input);
// 解析输入转换为链表
for (size_t i = 0; i < strlen(input); i += 4) {
int part = std::stoi(input.substr(i, 4));
if (input[i] == '-') {
sign = -1;
}
num1.add(sign * part);
}
std::cout << "请输入第二个100位以内长整数,每四位一组,以逗号分隔: ";
std::getline(std::cin, input);
// 同样解析输入
for (size_t i = 0; i < strlen(input); i += 4) {
part = std::stoi(input.substr(i, 4));
num2.add(part);
}
// 运算并输出结果
std::cout << "加法结果: " << num1.add(num2) << "\n";
std::cout << "减法结果: " << num1.subtract(num2) << "\n";
return 0;
}
```
阅读全文
相关推荐
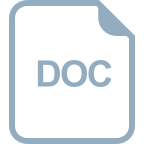
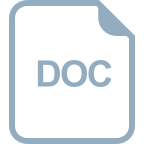
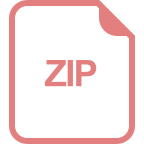
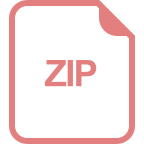
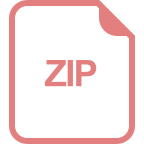
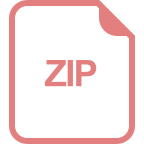
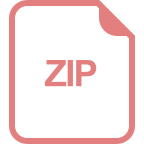
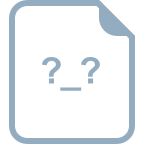
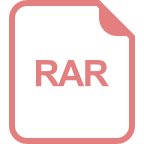
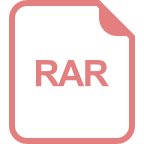
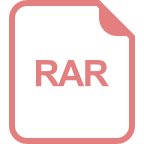
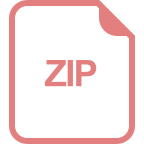
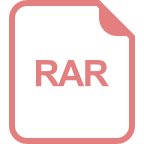