有个文件夹,里面有很多子文件夹,子文件夹里有很多文件。可能存在好几个子文件夹有文件相同,写个python脚本实现如果子文件夹里有文件相同,就合并这些文件夹里的文件放到其中一个文件夹里,并删除其余空文件夹
时间: 2023-04-01 11:04:50 浏览: 198
可以使用Python中的os和shutil模块来实现这个功能。首先,我们需要遍历文件夹中的所有子文件夹,找到其中有相同文件的子文件夹。然后,将这些子文件夹中的文件复制到其中一个文件夹中,并删除其余空文件夹。
以下是示例代码:
```python
import os
import shutil
# 遍历文件夹中的所有子文件夹
def find_duplicate_folders(root_folder):
folders = {}
for foldername, subfolders, filenames in os.walk(root_folder):
# 计算每个子文件夹中的文件的哈希值
file_hashes = {}
for filename in filenames:
filepath = os.path.join(foldername, filename)
with open(filepath, 'rb') as f:
file_hash = hash(f.read())
if file_hash in file_hashes:
# 如果哈希值相同,则说明有相同的文件
duplicate_folder = file_hashes[file_hash]
if duplicate_folder in folders:
# 如果已经找到过相同文件的子文件夹,则将当前子文件夹中的文件复制到那个子文件夹中
for filename in filenames:
src_file = os.path.join(foldername, filename)
dst_file = os.path.join(duplicate_folder, filename)
shutil.copy2(src_file, dst_file)
# 删除当前子文件夹
shutil.rmtree(foldername)
else:
# 如果还没有找到相同文件的子文件夹,则将当前子文件夹和那个子文件夹都记录下来
folders[foldername] = True
folders[duplicate_folder] = True
else:
file_hashes[file_hash] = foldername
# 测试代码
root_folder = 'path/to/root/folder'
find_duplicate_folders(root_folder)
```
注意,这个脚本只会合并有相同文件的子文件夹,如果有多个子文件夹中都有相同的文件,它们会被分别合并到不同的子文件夹中。如果需要将它们合并到同一个子文件夹中,可以在复制文件时给它们加上不同的前缀或后缀。
相关推荐
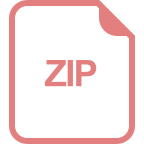
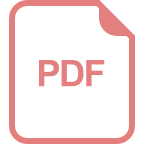
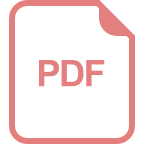















