springboot后端统一限制文件类型和大小
时间: 2023-07-03 12:30:25 浏览: 183
要在Spring Boot后端进行统一限制文件类型和大小,可以使用Spring Boot的MultipartFile类,通过在控制器中添加自定义注解并结合Spring Boot的拦截器实现。
首先,可以定义一个自定义注解,例如@FileValidator,用于限制文件类型和大小。在注解中添加两个属性,一个是允许上传的文件类型(MIME类型),另一个是允许上传的文件最大大小。
然后,可以编写一个拦截器,用于拦截文件上传请求,并在拦截器中进行文件类型和大小的验证。如果验证不通过,则可以返回一个自定义的错误信息。
最后,在控制器中使用@FileValidator注解对文件上传进行限制,即可实现Spring Boot后端统一限制文件类型和大小的功能。
以下是一个示例代码:
定义自定义注解:
```java
@Target(ElementType.PARAMETER)
@Retention(RetentionPolicy.RUNTIME)
public @interface FileValidator {
String[] allowedTypes() default {}; // 允许上传的文件类型
long maxSize() default 0; // 允许上传的文件最大大小
}
```
编写拦截器:
```java
@Component
public class FileUploadInterceptor implements HandlerInterceptor {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
if (handler instanceof HandlerMethod) {
HandlerMethod handlerMethod = (HandlerMethod) handler;
MethodParameter[] methodParameters = handlerMethod.getMethodParameters();
for (MethodParameter parameter : methodParameters) {
if (parameter.hasParameterAnnotation(FileValidator.class)) {
MultipartFile file = ((MultipartHttpServletRequest) request).getFile(parameter.getParameterName());
if (file == null) {
throw new CustomException("文件不能为空");
}
FileValidator fileValidator = parameter.getParameterAnnotation(FileValidator.class);
if (fileValidator.allowedTypes().length > 0 && !Arrays.asList(fileValidator.allowedTypes()).contains(file.getContentType())) {
throw new CustomException("文件类型不允许");
}
if (fileValidator.maxSize() > 0 && file.getSize() > fileValidator.maxSize()) {
throw new CustomException("文件大小超出限制");
}
}
}
}
return true;
}
}
```
在控制器中使用@FileValidator注解:
```java
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") @FileValidator(allowedTypes = {"image/jpeg", "image/png"}, maxSize = 10485760) MultipartFile file) {
// 处理上传文件
}
```
上面的示例中,@FileValidator注解用于限制文件类型为image/jpeg或image/png,大小不超过10M。拦截器会在请求到达控制器之前对文件进行验证,如果不符合要求,则会抛出CustomException异常。控制器中的@RequestParam注解用于接收上传的文件,并结合@FileValidator注解进行限制。
阅读全文
相关推荐
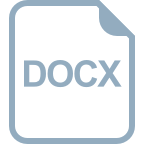
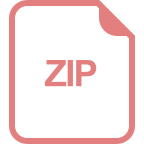
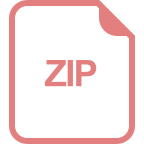
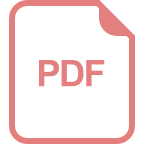
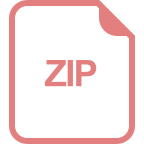