输入任何大小的迷宫数据,使用非递归方法(堆栈或队列)找到走出迷宫的路径,并输出路径;
时间: 2023-05-17 12:03:02 浏览: 125
好的,我可以回答这个问题。以下是一个使用队列的非递归方法来找到迷宫路径的示例代码:
```python
from collections import deque
def find_path(maze):
# 定义起点和终点
start = (0, 0)
end = (len(maze)-1, len(maze[0])-1)
# 定义方向
directions = [(0, 1), (0, -1), (1, 0), (-1, 0)]
# 定义队列
queue = deque()
queue.append(start)
# 定义记录路径的字典
path = {start: None}
# 开始搜索
while queue:
curr = queue.popleft()
if curr == end:
break
for d in directions:
next_pos = (curr[0]+d[0], curr[1]+d[1])
if 0 <= next_pos[0] < len(maze) and 0 <= next_pos[1] < len(maze[0]) and maze[next_pos[0]][next_pos[1]] == 0 and next_pos not in path:
queue.append(next_pos)
path[next_pos] = curr
# 构建路径
if end not in path:
return None
curr = end
path_list = []
while curr:
path_list.append(curr)
curr = path[curr]
path_list.reverse()
return path_list
```
这个函数接受一个二维数组作为输入,其中0表示可以通过的路径,1表示墙壁。它返回一个路径列表,其中包含从起点到终点的所有坐标。如果没有找到路径,则返回None。
阅读全文
相关推荐


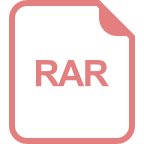
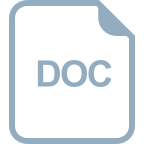







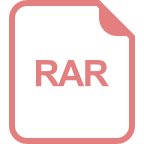



