c语言 链栈实现数制的转换
时间: 2023-11-02 20:00:53 浏览: 225
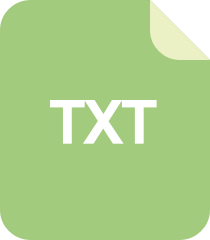
用c语言中栈实现数制转换

以下是一个C语言的链栈实现数制转换的示例代码:
```
#include <stdio.h>
#include <stdlib.h>
#define STACK_INIT_SIZE 100
#define STACK_INCREMENT 10
typedef struct StackNode {
int data;
struct StackNode *next;
} StackNode, *LinkStackPtr;
typedef struct {
LinkStackPtr top;
int stack_size;
} LinkStack;
void InitStack(LinkStack *S) {
S->top = NULL;
S->stack_size = 0;
}
int StackEmpty(LinkStack *S) {
if (S->top == NULL) {
return 1;
} else {
return 0;
}
}
void Push(LinkStack *S, int e) {
StackNode *p = (StackNode *)malloc(sizeof(StackNode));
p->data = e;
p->next = S->top;
S->top = p;
S->stack_size++;
}
int Pop(LinkStack *S, int *e) {
if (StackEmpty(S)) {
return 0;
}
StackNode *p = S->top;
*e = p->data;
S->top = p->next;
free(p);
S->stack_size--;
return 1;
}
int GetTop(LinkStack *S, int *e) {
if (StackEmpty(S)) {
return 0;
}
*e = S->top->data;
return 1;
}
void Convert(int n, int radix) {
LinkStack S;
InitStack(&S);
while (n != 0) {
Push(&S, n % radix);
n = n / radix;
}
while (!StackEmpty(&S)) {
int e;
Pop(&S, &e);
if (e < 10) {
printf("%d", e);
} else {
printf("%c", e - 10 + 'A');
}
}
printf("\n");
}
int main() {
int n, radix;
printf("Enter an integer: ");
scanf("%d", &n);
printf("Enter the radix: ");
scanf("%d", &radix);
printf("The converted number is: ");
Convert(n, radix);
return 0;
}
```
在这个示例代码中,我们首先定义了一个链栈的数据结构,包括链栈节点的数据结构和链栈的数据结构。然后我们实现了链栈的基本操作,包括初始化、判断是否为空、入栈、出栈、获取栈顶元素等操作。
最后,我们使用链栈实现了数制转换的功能。具体来说,我们将需要转换的整数不断除以进制数,将得到的余数入栈。然后我们不断出栈,将出栈的元素转换为对应的进制数的字符,最终输出结果。在输出结果时,如果余数小于10,直接输出数字;如果余数大于等于10,转换为对应的大写字母输出。
阅读全文
相关推荐
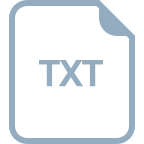

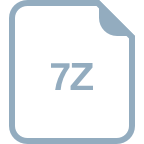
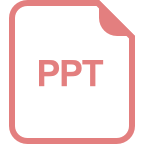
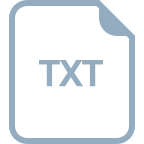
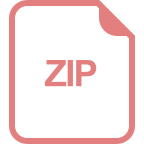
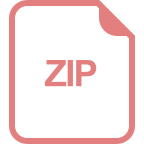
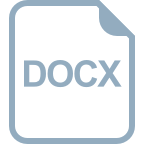
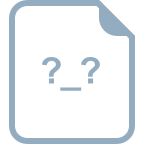
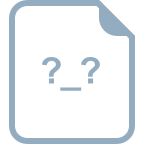