栈是一种“先进后出”的数据结构,它具有如下操作: push,将元素压入栈中 pop,将栈顶元素弹出 size,返回栈中元素个数 empty,判断栈是否为空 现在要求利用标准库中的vector类实现栈,其声明如下: template<typename T> class Mystack{ private: vector<T> elems; public: void push(T&& e); void pop(T& e); int size() const; bool empty() const; }; 请实现该类,并通过相应的测试程序。 测试程序: #include<vector> #include<iostream> using namespace std; template<typename T> class Mystack{ private: vector<T> elems; public: void push(T&& e); void pop(T& e); int size() const; bool empty() const; }; /* 请在这里填写答案 */ int main() { Mystack<int> s; s.push(10); s.push(20); s.push(30); cout<<s.size()<<endl; while(!s.empty()) { int e; s.pop(e); cout<<e<<endl; } return 0; } 输入样例: 输出样例: 3 30 20 10
时间: 2023-06-24 15:08:21 浏览: 97
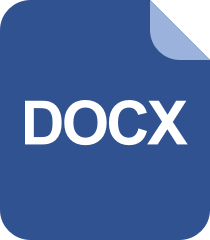
数据结构:图解链表,用链表实现栈的Pop和Push(c语言版)
参考实现:
```
#include<vector>
#include<iostream>
using namespace std;
template<typename T>
class Mystack {
private:
vector<T> elems;
public:
void push(T&& e) {
elems.push_back(e);
}
void pop(T& e) {
if (!elems.empty()) {
e = elems.back();
elems.pop_back();
}
else {
cout << "Stack is empty!" << endl;
}
}
int size() const {
return elems.size();
}
bool empty() const {
return elems.empty();
}
};
int main() {
Mystack<int> s;
s.push(10);
s.push(20);
s.push(30);
cout << s.size() << endl;
while (!s.empty()) {
int e;
s.pop(e);
cout << e << endl;
}
return 0;
}
```
阅读全文
相关推荐
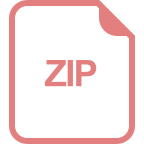
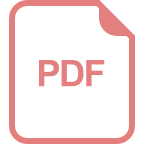















