写出三种工厂模式的代码示例
时间: 2024-06-07 19:02:55 浏览: 14
当我们需要创建对象时,使用工厂模式可以将对象的创建与使用分离,这样能够提高代码的可读性和可维护性。常见的工厂模式有三种:简单工厂模式、工厂方法模式和抽象工厂模式。下面是三种工厂模式的代码示例:
1. 简单工厂模式:
```python
class Product:
def operate(self):
pass
class ProductA(Product):
def operate(self):
print('ProductA operation')
class ProductB(Product):
def operate(self):
print('ProductB operation')
class SimpleFactory:
def create_product(self, product_type):
if product_type == 'A':
return ProductA()
elif product_type == 'B':
return ProductB()
else:
raise ValueError(f'Invalid product type: {product_type}')
# 使用简单工厂创建对象
factory = SimpleFactory()
product_a = factory.create_product('A')
product_b = factory.create_product('B')
product_a.operate() # Output: "ProductA operation"
product_b.operate() # Output: "ProductB operation"
```
2. 工厂方法模式:
```python
class Product:
def operate(self):
pass
class ProductA(Product):
def operate(self):
print('ProductA operation')
class ProductB(Product):
def operate(self):
print('ProductB operation')
class Factory:
def create_product(self):
pass
class FactoryA(Factory):
def create_product(self):
return ProductA()
class FactoryB(Factory):
def create_product(self):
return ProductB()
# 使用工厂方法创建对象
factory_a = FactoryA()
product_a = factory_a.create_product()
factory_b = FactoryB()
product_b = factory_b.create_product()
product_a.operate() # Output: "ProductA operation"
product_b.operate() # Output: "ProductB operation"
```
3. 抽象工厂模式:
```python
class AbstractFactory:
def create_product_a(self):
pass
def create_product_b(self):
pass
class ConcreteFactory1(AbstractFactory):
def create_product_a(self):
return ConcreteProductA1()
def create_product_b(self):
return ConcreteProductB1()
class ConcreteFactory2(AbstractFactory):
def create_product_a(self):
return ConcreteProductA2()
def create_product_b(self):
return ConcreteProductB2()
class AbstractProductA:
def operate(self):
pass
class ConcreteProductA1(AbstractProductA):
def operate(self):
print('ConcreteProductA1 operation')
class ConcreteProductA2(AbstractProductA):
def operate(self):
print('ConcreteProductA2 operation')
class AbstractProductB:
def operate(self):
pass
class ConcreteProductB1(AbstractProductB):
def operate(self):
print('ConcreteProductB1 operation')
class ConcreteProductB2(AbstractProductB):
def operate(self):
print('ConcreteProductB2 operation')
# 使用抽象工厂创建对象
factory1 = ConcreteFactory1()
product_a1 = factory1.create_product_a()
product_b1 = factory1.create_product_b()
product_a1.operate() # Output: "ConcreteProductA1 operation"
product_b1.operate() # Output: "ConcreteProductB1 operation"
factory2 = ConcreteFactory2()
product_a2 = factory2.create_product_a()
product_b2 = factory2.create_product_b()
product_a2.operate() # Output: "ConcreteProductA2 operation"
product_b2.operate() # Output: "ConcreteProductB2 operation"
```
相关推荐
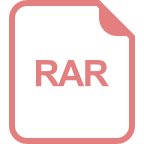
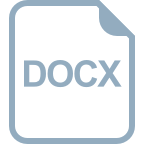
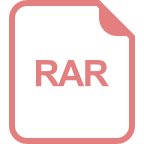















