遗传算法解决多维背包问题
时间: 2024-03-19 07:38:40 浏览: 21
遗传算法是一种基于生物进化原理的优化算法,常用于解决多维背包问题等组合优化问题。下面是遗传算法解决多维背包问题的基本步骤:
1. 初始化种群:随机生成一组初始解,每个解表示一组物品的选择情况。
2. 适应度评估:根据每个解的适应度函数,计算每个解的适应度值,用于评估解的优劣程度。
3. 选择操作:根据适应度值,采用选择算子选择一部分优秀的解作为父代。
4. 交叉操作:对选出的父代进行交叉操作,生成新的子代。交叉操作可以通过交换染色体片段、单点交叉等方式进行。
5. 变异操作:对子代进行变异操作,引入一定的随机性。变异操作可以通过改变染色体中的基因值或位置等方式进行。
6. 替换操作:将子代替换掉部分父代,形成新一代种群。
7. 终止条件判断:判断是否满足终止条件,如达到最大迭代次数或找到满意的解等。
8. 返回最优解:返回最优解作为问题的解决方案。
相关问题
遗传算法解决多维背包问题代码
遗传算法是一种模拟自然进化过程的优化算法,可以用于解决多维背包问题。下面是一个简单的遗传算法解决多维背包问题的代码示例:
```python
import random
# 背包问题的物品列表,每个物品有重量和价值两个属性
items = [
{"weight": 2, "value": 3},
{"weight": 3, "value": 4},
{"weight": 4, "value": 5},
# 其他物品...
]
# 背包的容量限制
capacity = 7
# 遗传算法的参数设置
population_size = 50 # 种群大小
mutation_rate = 0.01 # 变异率
generations = 100 # 迭代次数
# 初始化种群
def initialize_population():
population = []
for _ in range(population_size):
chromosome = [random.randint(0, 1) for _ in range(len(items))]
population.append(chromosome)
return population
# 计算染色体的适应度(总价值)
def fitness(chromosome):
total_weight = 0
total_value = 0
for i in range(len(chromosome)):
if chromosome[i] == 1:
total_weight += items[i]["weight"]
total_value += items[i]["value"]
if total_weight > capacity:
total_value = 0
return total_value
# 选择操作(轮盘赌选择)
def selection(population):
fitness_values = [fitness(chromosome) for chromosome in population]
total_fitness = sum(fitness_values)
probabilities = [fitness_value / total_fitness for fitness_value in fitness_values]
selected_indices = random.choices(range(len(population)), probabilities, k=2)
return [population[index] for index in selected_indices]
# 交叉操作(单点交叉)
def crossover(parent1, parent2):
crossover_point = random.randint(1, len(parent1) - 1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
# 变异操作(随机翻转一个基因位)
def mutation(chromosome):
mutated_chromosome = chromosome[:]
gene_index = random.randint(0, len(chromosome) - 1)
mutated_chromosome[gene_index] = 1 - mutated_chromosome[gene_index]
return mutated_chromosome
# 遗传算法主循环
def genetic_algorithm():
population = initialize_population()
for _ in range(generations):
new_population = []
while len(new_population) < population_size:
parent1, parent2 = selection(population)
child1, child2 = crossover(parent1, parent2)
if random.random() < mutation_rate:
child1 = mutation(child1)
if random.random() < mutation_rate:
child2 = mutation(child2)
new_population.append(child1)
new_population.append(child2)
population = new_population
best_chromosome = max(population, key=fitness)
best_fitness = fitness(best_chromosome)
return best_chromosome, best_fitness
# 运行遗传算法并输出结果
best_chromosome, best_fitness = genetic_algorithm()
print("最优解:", best_chromosome)
print("最优解的适应度:", best_fitness)
```
这段代码实现了一个简单的遗传算法来解决多维背包问题。其中,`items`列表定义了背包中的物品,`capacity`表示背包的容量限制。遗传算法的参数设置包括种群大小、变异率和迭代次数。代码中的`initialize_population`函数用于初始化种群,`fitness`函数计算染色体的适应度,`selection`函数进行选择操作,`crossover`函数进行交叉操作,`mutation`函数进行变异操作。最后,`genetic_algorithm`函数是遗传算法的主循环,通过迭代生成新的种群,并找到适应度最高的染色体作为最优解。
蚁群算法解决多维背包问题
蚁群算法是一种模拟蚂蚁觅食行为的启发式优化算法,可以用于解决多维背包问题。在多维背包问题中,每个物品有多个属性,如重量、体积、价值等,而背包有一定的容量限制。目标是选择一组物品放入背包中,使得总体价值最大化,同时满足容量限制。
蚁群算法的基本思想是通过模拟蚂蚁在搜索空间中的行为,通过信息素的交流和挥发来引导搜索过程。对于多维背包问题,可以将每个蚂蚁看作是一个解决方案,它通过选择不同的物品来构建一个可行解。每个蚂蚁根据一定的启发式规则选择物品,并更新信息素。
具体而言,在蚁群算法中,可以将每个物品看作是一个节点,每个节点上有一个信息素值表示该物品被选择的概率。蚂蚁根据信息素值和启发式规则选择下一个物品,并更新路径上经过的物品的信息素值。通过多次迭代,信息素会逐渐集中在较优解路径上,从而得到一个较优的解。
需要注意的是,蚁群算法虽然可以用于解决多维背包问题,但是在实际应用中可能需要进行一些问题的转化和适应性调整。例如,需要将问题转化为离散化的形式,将物品的属性以及背包容量量化为一定的范围。
总结起来,蚁群算法可以用来解决多维背包问题,通过模拟蚂蚁觅食行为来搜索最优解。但在具体应用中需要根据问题的特点进行适当的调整和转化。
相关推荐
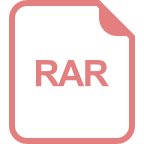
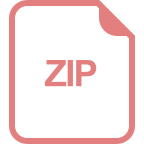


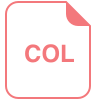
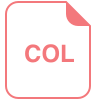








