帮我用c++写一个类 包含各种重载运算符,友元函数,虚函数,以及使用,写出完整代码
时间: 2023-12-04 12:01:36 浏览: 35
好的,下面是一个包含各种重载运算符、友元函数、虚函数的类的完整 C++ 代码示例:
```cpp
#include <iostream>
using namespace std;
class Shape {
public:
virtual ~Shape() {}
virtual double area() const = 0;
virtual double perimeter() const = 0;
virtual void print() const = 0;
// 重载运算符
bool operator<(const Shape& rhs) const {
return area() < rhs.area();
}
bool operator>(const Shape& rhs) const {
return area() > rhs.area();
}
// 友元函数
friend ostream& operator<<(ostream& os, const Shape& shape) {
os << "Area: " << shape.area() << ", Perimeter: " << shape.perimeter() << endl;
return os;
}
};
class Rectangle : public Shape {
public:
Rectangle(double w, double h) : width(w), height(h) {}
virtual ~Rectangle() {}
virtual double area() const {
return width * height;
}
virtual double perimeter() const {
return 2 * (width + height);
}
virtual void print() const {
cout << "Rectangle: ";
cout << "Width = " << width << ", Height = " << height << endl;
}
private:
double width;
double height;
};
class Circle : public Shape {
public:
Circle(double r) : radius(r) {}
virtual ~Circle() {}
virtual double area() const {
return 3.14 * radius * radius;
}
virtual double perimeter() const {
return 2 * 3.14 * radius;
}
virtual void print() const {
cout << "Circle: ";
cout << "Radius = " << radius << endl;
}
private:
double radius;
};
int main() {
Shape* shapes[2];
shapes[0] = new Rectangle(3, 4);
shapes[1] = new Circle(5);
for (int i = 0; i < 2; i++) {
shapes[i]->print();
cout << *shapes[i];
}
if (*shapes[0] < *shapes[1]) {
cout << "Rectangle is smaller than Circle." << endl;
} else {
cout << "Circle is smaller than Rectangle." << endl;
}
delete shapes[0];
delete shapes[1];
return 0;
}
```
在这段代码中,我们定义了一个抽象类 `Shape`,它包含了纯虚函数 `area()`、`perimeter()` 和 `print()`。这个抽象类还重载了运算符 `<` 和 `>`,并定义了一个友元函数 `operator<<`,用于输出类对象的信息。
我们还定义了两个派生类 `Rectangle` 和 `Circle`,它们分别实现了 `Shape` 的纯虚函数。这两个类还重载了运算符 `<` 和 `>`,但是它们并没有定义友元函数。
在 `main()` 函数中,我们创建了两个形状对象(一个矩形和一个圆),并将它们存储在一个数组中。然后,我们循环遍历这些形状对象,并打印它们的信息。最后,我们使用重载的 `<` 运算符比较这两个形状对象的大小,并输出比较结果。
注意,由于 `Shape` 是一个抽象类,我们不能直接创建它的对象。因此,我们使用指针来存储它的派生类对象。在删除这些对象时,我们需要使用 `delete` 运算符,以避免内存泄漏。
相关推荐
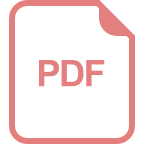
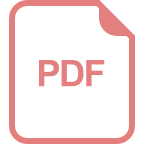














