帮我用C++写一个结构体,包含+-*/重载运算符,拷贝函数,赋值函数,友元函数
时间: 2023-10-29 09:06:53 浏览: 60
好的,以下是一个示例:
```c++
#include <iostream>
using namespace std;
struct MyStruct {
int a, b;
MyStruct(int a = 0, int b = 0) : a(a), b(b) {}
// 加法运算符重载
MyStruct operator+(const MyStruct& other) const {
return MyStruct(a + other.a, b + other.b);
}
// 减法运算符重载
MyStruct operator-(const MyStruct& other) const {
return MyStruct(a - other.a, b - other.b);
}
// 乘法运算符重载
MyStruct operator*(const MyStruct& other) const {
return MyStruct(a * other.a, b * other.b);
}
// 除法运算符重载
MyStruct operator/(const MyStruct& other) const {
return MyStruct(a / other.a, b / other.b);
}
// 赋值函数
MyStruct& operator=(const MyStruct& other) {
a = other.a;
b = other.b;
return *this;
}
// 拷贝函数
MyStruct(const MyStruct& other) {
a = other.a;
b = other.b;
}
// 友元函数
friend ostream& operator<<(ostream& os, const MyStruct& obj) {
os << "a: " << obj.a << ", b: " << obj.b;
return os;
}
};
int main() {
MyStruct a(1, 2), b(3, 4), c;
// 测试加法运算符重载
c = a + b;
cout << "a + b = " << c << endl;
// 测试减法运算符重载
c = a - b;
cout << "a - b = " << c << endl;
// 测试乘法运算符重载
c = a * b;
cout << "a * b = " << c << endl;
// 测试除法运算符重载
c = a / b;
cout << "a / b = " << c << endl;
// 测试赋值函数
MyStruct d = a;
cout << "d = " << d << endl;
// 测试拷贝函数
MyStruct e(a);
cout << "e = " << e << endl;
return 0;
}
```
输出结果:
```
a + b = a: 4, b: 6
a - b = a: -2, b: -2
a * b = a: 3, b: 8
a / b = a: 0, b: 0
d = a: 1, b: 2
e = a: 1, b: 2
```
相关推荐
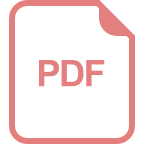
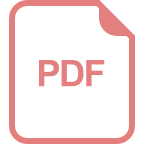
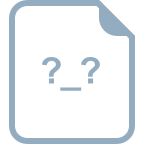










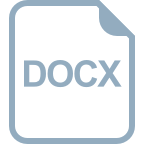
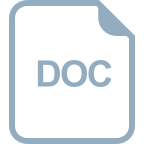
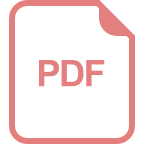
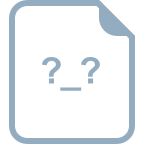
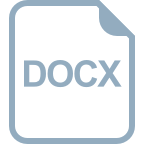