YOLOv5转onnx GPU
时间: 2023-07-08 12:09:41 浏览: 90
您好,这是一个IT类问题。关于将YOLOv5转换为ONNX格式并在GPU上运行的问题,您可以按照以下步骤操作:
1. 安装ONNX:使用pip命令安装ONNX,例如:pip install onnx
2. 下载YOLOv5模型:从YOLO官方网站下载预训练的YOLOv5模型。
3. 转换模型:使用torch.onnx.export函数将PyTorch模型转换为ONNX格式。例如:
```
import torch
from models.experimental import attempt_load
# 加载YOLOv5预训练模型
weights = 'yolov5s.pt'
model = attempt_load(weights, map_location=torch.device('cpu'))
# 导出模型为ONNX格式
input_shape = (3, 640, 640)
input_names = ['input']
output_names = ['output']
dynamic_axes = {'input': {0: 'batch_size'}, 'output': {0: 'batch_size'}}
onnx_path = 'yolov5s.onnx'
torch.onnx.export(model, torch.randn(1, *input_shape), onnx_path, verbose=True,
input_names=input_names, output_names=output_names, dynamic_axes=dynamic_axes)
```
4. 加载ONNX模型:使用onnxruntime库加载ONNX模型。例如:
```
import onnxruntime
# 加载ONNX模型
onnx_path = 'yolov5s.onnx'
ort_session = onnxruntime.InferenceSession(onnx_path)
```
5. 运行模型:使用导出的ONNX模型在GPU上运行推理。例如:
```
import cv2
import numpy as np
# 加载测试图像
image_path = 'test.jpg'
image = cv2.imread(image_path)
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
image = cv2.resize(image, (640, 640))
image = np.expand_dims(image.transpose((2, 0, 1)), axis=0)
# 在GPU上运行推理
input_name = ort_session.get_inputs()[0].name
output_name = ort_session.get_outputs()[0].name
ort_inputs = {input_name: image}
ort_outputs = ort_session.run([output_name], ort_inputs)
# 解析输出结果
output = ort_outputs[0]
print(output.shape)
```
注意:在运行ONNX模型之前,请确保您已经安装了适当的GPU驱动程序和CUDA工具包,并在代码中指定使用GPU设备。
相关推荐
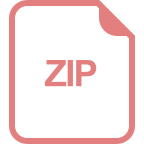
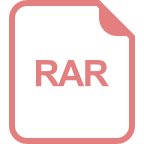














