在Unity中如何使用代码计算不规则物体的体积,物体内部有空洞
时间: 2024-03-06 21:49:55 浏览: 19
要计算具有空洞的不规则物体的体积,可以使用Unity的Mesh API来实现。具体步骤如下:
1. 获取物体的Mesh组件,如果需要计算多个Mesh组件的总体积,则需要将它们合并成一个Mesh。
```csharp
MeshFilter[] meshFilters = gameObject.GetComponentsInChildren<MeshFilter>();
CombineInstance[] combine = new CombineInstance[meshFilters.Length];
for (int i = 0; i < meshFilters.Length; i++)
{
combine[i].mesh = meshFilters[i].sharedMesh;
combine[i].transform = meshFilters[i].transform.localToWorldMatrix;
}
Mesh mesh = new Mesh();
mesh.CombineMeshes(combine, true);
```
2. 使用Mesh API中的ComputeBounds方法获取物体的包围盒。
```csharp
Bounds bounds = mesh.bounds;
```
3. 创建一个Raycast射线,从包围盒的最小点开始向物体内部发射,计算射线与物体内部的交点。
```csharp
Vector3 rayOrigin = bounds.min;
float step = bounds.size.magnitude / 100f;
float voxelVolume = step * step * step;
int count = 0;
for (float x = bounds.min.x; x < bounds.max.x; x += step)
{
for (float y = bounds.min.y; y < bounds.max.y; y += step)
{
for (float z = bounds.min.z; z < bounds.max.z; z += step)
{
Vector3 voxel = new Vector3(x, y, z);
Ray ray = new Ray(voxel, Vector3.right);
if (mesh.bounds.IntersectRay(ray))
{
count++;
}
}
}
}
float volume = (float)count * voxelVolume;
```
4. 计算所有体素的体积之和,即为物体的体积。
需要注意的是,这种方法只适用于简单的不规则物体。如果物体非常复杂,可能需要使用更高级的算法来计算体积。此外,该方法还可能存在误差,因为它使用了离散的体素来近似物体的体积。
相关推荐
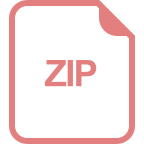
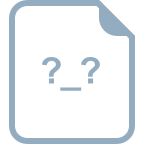














