Linux C code demo on how IPv6 accesses IPv4 addresses
时间: 2024-05-14 14:17:37 浏览: 117
Here is some sample C code to demonstrate how IPv6 can access IPv4 addresses:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <errno.h>
int main()
{
struct sockaddr_in6 addr;
int sockfd;
// create a socket
sockfd = socket(AF_INET6, SOCK_STREAM, 0);
if (sockfd < 0) {
perror("socket error");
exit(1);
}
// set the address
memset(&addr, 0, sizeof(addr));
addr.sin6_family = AF_INET6;
addr.sin6_port = htons(80);
inet_pton(AF_INET, "192.168.1.1", &addr.sin6_addr);
// connect to the server
if (connect(sockfd, (struct sockaddr*)&addr, sizeof(addr)) < 0) {
perror("connect error");
exit(1);
}
printf("Connected to server\n");
close(sockfd);
return 0;
}
```
In this example, we create a socket using the `socket()` function with the `AF_INET6` address family. We then set the address using the `inet_pton()` function to convert an IPv4 address string to a binary format that can be used with IPv6. Finally, we connect to the server using the `connect()` function and close the socket when we are done.
Note that this code assumes that the server we are connecting to is reachable through an IPv6 address that can access the IPv4 address we are using. In practice, this may require additional configuration on the server side.
阅读全文
相关推荐
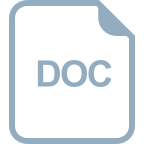
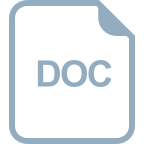
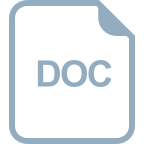


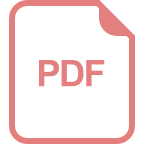
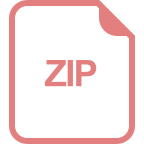
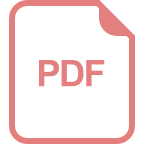
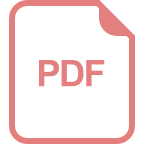
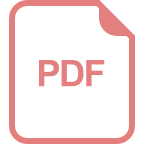
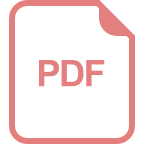
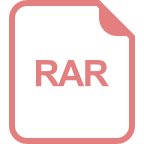
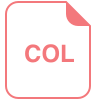





