MATLAB Path and Code Reusability: Path Management Strategies to Promote Code Reusability and Modularization, Eliminating Code Duplication
发布时间: 2024-09-14 14:08:36 阅读量: 26 订阅数: 29 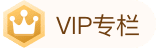
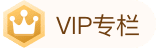
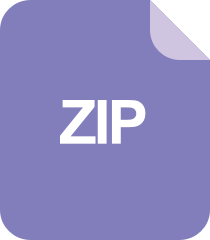
react_props_reusability:一个演示如何制作可重用道具的演示
# 1. Introduction to MATLAB Path Management
The MATLAB path is a list of directories that MATLAB searches for functions, data files, and other resources. Effective path management is crucial for organizing code, promoting code reuse, and preventing code duplication.
MATLAB provides a set of functions and mechanisms to manage the path, including:
***addpath() and rmpath() functions:** Used to add and remove directories from the path.
***savepath() and restorepath() functions:** Used to save and restore path settings.
# 2. Path Management Strategies
### 2.1 Adding and Removing Directories from the Path
#### 2.1.1 addpath() and rmpath() Functions
***addpath() function:** Adds specified folders or files to the MATLAB path.
```
addpath('path/to/folder');
```
* Parameter explanation:
* 'path/to/folder': The folder or file to be added to the path.
***rmpath() function:** Removes specified folders or files from the MATLAB path.
```
rmpath('path/to/folder');
```
* Parameter explanation:
* 'path/to/folder': The folder or file to be removed from the path.
#### 2.1.2 savepath() and restorepath() Functions
***savepath() function:** Saves the current MATLAB path to a file.
```
savepath('path_file.mat');
```
* Parameter explanation:
* 'path_file.mat': The filename to save the path.
***restorepath() function:** Restores the MATLAB path from a file.
```
restorepath('path_file.mat');
```
* Parameter explanation:
* 'path_file.mat': The filename from which to restore the path.
### 2.2 Path Search Order
#### 2.2.1 path() Function
***path() function:** Returns a list of folders and files in the MATLAB path.
```
path_list = path();
```
* Parameter explanation:
* path_list: A string array containing the list of folders and files in the MATLAB path.
MATLAB searches paths in the following order:
1. Current folder
2. Folders and files in the MATLAB path
3. Folders and files in the MATLAB installation directory
#### 2.2.2 pathdef.m File
***pathdef.m ***
***
***
***
***
***
***
```
pathcache(true); % Enable path caching
pathcache(false); % Disable path caching
```
* Parameter explanation:
* true/false: Enable or disable path caching.
#### 2.3.2 genpath() Function
***genpath() function:** Generates a path list that includes the specified folder and all its subfolders.
```
path_list = genpath('path/to/folder');
```
* Parameter explanation:
* 'path/to/folder': The folder for which to generate the path list.
* This function can be used to accelerate adding paths, as MATLAB can process the entire folder structure in one go.
# 3. Techniques for Code Reuse
### 3.1 Functions and Scripts
#### 3.1.1 Function Definition and Invocation
Functions are reusable blocks of code in MATLAB designed to perform specific tasks. Function definitions use the `function` keyword, followed by the function name, input parameters (if any), and output parameters (if any).
```matlab
function [output1, output2] = myFunction(input1, input2)
% Code block
end
```
To invoke a function, use the function name followed by any input parameters (if any).
```matlab
[result1, result2] = myFunction(arg1, arg2);
```
**Code Logic Analysis:**
* The function `myFunction` defines two output parameters, `output1` and `output2`, and two input parameters, `input1` and `input2`.
* The
0
0
相关推荐







