【MATLAB Path Management Tips】: A Trick to Solve Path Issues and Enhance Code Running Efficiency
发布时间: 2024-09-14 13:37:20 阅读量: 44 订阅数: 29 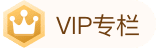
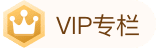
# MATLAB Path Management: A Comprehensive Guide to Streamline Code Efficiency
## 1. Overview of MATLAB Path Management
MATLAB path management is the process of handling the directories in which MATLAB searches for files and functions. It is crucial for optimizing MATLAB's performance and preventing errors. The MATLAB path is a set of directories where MATLAB looks for files and functions. Effective path management allows users to speed up loading times, simplify file access, and enhance the overall MATLAB experience.
## 2. Theoretical Foundations of MATLAB Path Management
**2.1 The Concept and Classification of Paths**
The MATLAB path is an ordered list of locations where MATLAB searches for files (such as functions, data files, and scripts). Paths can be categorized into two types:
- **User paths:** User-defined paths for storing user-specific files.
- **System paths:** Predefined paths included in the MATLAB installation directory for housing core functions and toolboxes.
**2.2 Path Search Mechanism**
When MATLAB executes a command, it searches the paths in the following order:
1. Current directory
2. User paths
3. System paths
If MATLAB cannot find a file in these paths, it will return an error.
**2.3 Best Practices for Path Management**
Efficient path management is essential for MATLAB's performance and reliability. Here are some best practices:
- **Keep paths concise:** Only include necessary paths to avoid lengthy search times.
- **Use relative paths:** Relative paths are relative to the current directory and help maintain portability.
- **Avoid path conflicts:** Ensure paths do not point to multiple copies of the same file or directory.
- **Utilize path caching:** Enabling path caching can accelerate MATLAB's file loading speed.
- **Use version control:** For collaborative work, use version control systems (e.g., Git) to manage path changes.
**Code Examples:**
```matlab
% Add user path
addpath('my_path');
% Set default path
setpref('MATLAB','defaultPath','my_path');
% Find file dependencies
findDependencies('my_function.m');
```
**Logical Analysis:**
- The `addpath` function adds the specified path to the user path.
- The `setpref` function sets MATLAB preference settings, including the default path.
- The `findDependencies` function locates and displays the file dependencies of the specified function.
## 3. Practical Tips for MATLAB Path Management
### 3.1 Adding and Removing Paths
**Adding Paths**
There are several methods to add paths in MATLAB:
***Using the addpath function:**
```
addpath('path/to/directory')
```
***Using the pathtool GUI:**
1. Type `pathtool` in the MATLAB command window.
2. In the Pathtool window that appears, click the "Add Folder" button.
3. Select the folder you want to add.
***Using the setpref function:**
```
setpref('pathdef', 'MATLAB', {'path/to/directory', 'path/to/another/directory'});
```
**Removing Paths**
Here are the methods to remove paths in MATLAB:
***Using the rmpath function:**
```
rmpath('path/to/directory')
```
***Using the pathtool GUI:**
1. Type `pathtool` in the MATLAB command window.
2. In the Pathtool window that appears, select the path to be deleted.
3. Click the "Remove" button.
***Using the setpref function:**
```
setpref('pathdef', 'MATLAB', {'path/to/directory'});
```
### 3.2 Setting a Default Path
The default path in MATLAB is the one that is automatically loaded when MATLAB starts up. You can set a default path so that specific folders are automatically loaded every time MATLAB is launched.
**Setting a Default Path**
***Using the pathdef.m ***
*** `pathdef.m`.
2. Enter the following code in the file:
```
function p = pathdef
p = {'path/to/directory', 'path/to/another/directory'};
end
```
3. Save the file to the toolbox/local directory within the MATLAB installation directory.
***Using the setpref function:**
```
setpref('pathdef', 'MATLAB', {'path/to/directory', 'path/to/another/directory'});
```
### 3.3 Finding and Managing File Dependencies
In MATLAB, file dependencies refer to the relationship between one file and another file or function. Managing file dependencies is crucial for ensuring the correctness and maintainability of your code.
**Finding File Dependencies**
***Using the depfun function:**
```
depfun('function_name')
```
***Using the dependencygraph function:**
```
dependencygraph('function_name')
```
**Managing File Dependencies**
***Using the addpath function:**
Add the path of files containing dependencies to the MATLAB path.
***Using the savepath function:**
Save the current MATLAB path to a file for future loading.
***Using version control systems:**
Use version control systems (like Git or Subversion) to manage file dependencies, ensuring collaboration and traceability.
## 4. Advanced Applications in MATLAB Path Management
### 4.1 Path Caching and Accelerated Loading
The MATLAB path cache is a mechanism that stores path information to speed up subsequent loads. When MATLAB first loads paths, it stores the path information in the cache. For subsequent loads, MATLAB will first check the cache, and if the path information is available, it will load directly from the cache without searching the file system.
```
% Create a path cache
pathCache = pathdef;
% Load paths using the path cache
addpath(pathCache);
```
### 4.2 Path Version Control and Collaboration
In team collaboration, managing path version control and collaboration is essential. MATLAB offers a tool called Path Manager, which allows users to track and manage path changes. Path Manager is integrated into the MATLAB environment and provides a user-friendly interface for path management.
```
% Use Path Manager to track path changes
pathManager = pathmanager;
pathManager.trackChanges();
```
### 4.3 Path Optimization and Performance Enhancement
Optimizing path management can significantly improve MATLAB's loading speed and performance. Here are some tips for path optimization:
- **Avoid using relative paths:** Relative paths can increase search time as MATLAB needs to resolve the current directory to find files.
- **Use path caching:** As mentioned earlier, path caching can speed up path loading.
- **Use path prefixes:** Path prefixes allow MATLAB to prioritize the search in specific directories.
- **Use path exclusions:** Path exclusions allow MATLAB to ignore certain directories, reducing search time.
```
% Optimize paths using path prefixes
addpath(genpath('~/my_toolbox'));
% Optimize paths using path exclusions
addpath(genpath('~/my_toolbox'), '-exclude', '**/private');
```
## 5. Troubleshooting MATLAB Path Management
### 5.1 Common Path Issues and Solutions
**Issue: Cannot find function or file**
**Causes:**
- The path does not include the directory where the file or function is located.
- The filename or function name is misspelled.
- The file or function is corrupted or missing.
**Solutions:**
- Check if the path is correct and includes the directory where the file or function is located.
- Check if the filename or function name is spelled correctly.
- Redownload or reinstall the file or function.
**Issue: Path conflicts**
**Causes:**
- Two or more directories contain files or functions with the same name.
- Path order causes the incorrect version to be loaded first.
**Solutions:**
- Use the `which` command to find the path of the file or function.
- Adjust the path order to ensure the correct version is loaded first.
- Use the `rehash` command to update the path cache.
**Issue: Cyclic dependencies**
**Causes:**
- Two or more files or functions are mutually dependent, causing a circular reference.
**Solutions:**
- Reorganize files or functions to break the cyclic dependency.
- Use `addpath` and `rmpath` commands to dynamically manage paths.
**Issue: Path too long**
**Causes:**
- The path includes too many directories.
**Solutions:**
- Use the `pathtool` tool to manage paths and delete unnecessary directories.
- Use the `savepath` command to save the current path to a file and reload as needed.
### 5.2 Path Conflict and Dependency Management
**Path Conflicts**
Path conflicts occur when multiple directories contain files or functions with the same name. MATLAB will load the first file or function it finds in the path, which may result in loading the incorrect version, leading to unexpected behavior.
To resolve path conflicts, adjust the path order to prioritize the correct version. You can also use the `which` command to find the path of a file or function and use `addpath` and `rmpath` commands to dynamically manage paths.
**Dependency Management**
MATLAB files and functions often depend on other files and functions. When dependencies change, it may result in errors or unexpected behavior. To manage dependencies, use the following techniques:
- **Using the `pathdef.m` *** `pathdef.m` file defines the default paths for MATLAB on startup. Add dependencies to this file to ensure they are available in every session.
- **Using `addpath` and `rmpath` commands:** The `addpath` and `rmpath` commands allow for dynamic path management. Add dependencies to the path as needed and remove them when no longer required.
- **Using Version Control:** Version control systems (like Git) can track changes to files and functions. This helps manage dependencies and ensures consistency across different versions.
## 6. Summary of Best Practices for MATLAB Path Management
Following these best practices when managing MATLAB paths ensures efficient and error-free code execution:
***Keep paths short and well-organized:** Avoid adding unnecessary folders and use descriptive folder names to organize files.
***Use relative paths:** Relative paths are more portable and easier to manage in collaborative environments.
***Set default paths:** Add frequently used folders to the default path to streamline code execution and avoid manual path additions.
***Utilize path caching:** Enabling path caching can significantly speed up path searches, especially in large projects.
***Use version control:** Incorporate path information into version control systems to ensure collaboration among team members and code reproducibility.
***Regularly optimize paths:** As projects evolve, periodically review paths and remove folders that are no longer needed to enhance performance.
***Use path tools:** Leverage MATLAB's path tools (e.g., pathtool) to visualize and manage paths, simplifying path management tasks.
***Adhere to MATLAB path management guidelines:** Consult MATLAB documentation for the latest information on best practices and troubleshooting.
0
0
相关推荐








