MATLAB Path and Code Analysis: Optimizing Code Structure and Maintainability Using Path Information, Saying Goodbye to Code Chaos
发布时间: 2024-09-14 14:09:19 阅读量: 23 订阅数: 24 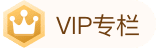
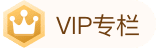
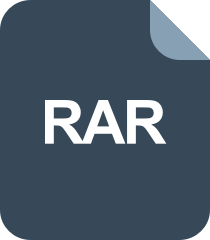
Java源码ssm框架的房屋租赁系统-合同-毕业设计论文-期末大作业.rar
# 1. Basic Concepts of MATLAB Path
A MATLAB path is a list of directories where MATLAB searches for functions, data files, and other resources. It is a semicolon-separated string where each directory is represented as a pathname. MATLAB starts searching from the first directory in the path and continues to the next directory if the resource is not found, and so on.
Paths can be absolute (starting from the root directory) or relative (starting from the current directory). MATLAB also supports path wildcards (such as `*` and `**`) for matching directories and files.
# 2.1 Adding and Removing Paths
### 2.1.1 addpath and rmpath Commands
In MATLAB, the `addpath` and `rmpath` commands are used for managing paths. The `addpath` command adds a directory to the current path, while the `rmpath` command removes a directory from the current path.
```
% Adding a directory to the current path
addpath('my_directory');
% Removing a directory from the current path
rmpath('my_directory');
```
### 2.1.2 Path Priority and Search Order
MATLAB searches for files in directories based on path priority. Path priority is determined by the order of directories in the path. It first searches the first directory in the path, then the subsequent directories.
```
% Setting the path
addpath('dir1');
addpath('dir2');
% Searching for a file
file = which('my_function.m');
% Displaying the result
disp(file);
```
In the example above, the `which` command will first search for `my_function.m` in `dir1`, and if not found, it will then search in `dir2`.
### Code Logic Analysis
```
% Adding a directory to the current path
addpath('my_directory');
% Parameter explanation:
% 'my_directory': The directory path to be added to the current path.
% Logical analysis:
The `addpath` command adds 'my_directory' to the current path. If 'my_directory' already exists in the current path, it will not be added again.
% Removing a directory from the current path
rmpath('my_directory');
% Parameter explanation:
% 'my_directory': The directory path to be removed from the current path.
% Logical analysis:
The `rmpath` command removes 'my_directory' from the current path. If 'my_directory' does not exist in the current path, no action will be taken.
```
# 3. Applying Paths in Code Analysis
### 3.1 Identifying Code Dependencies
**3.1.1 Dependency Analysis Tools**
MATLAB provides the `depfun` function to analyze dependencies in code. This function takes a function handle or function name as input and returns a structure containing information about other functions and files that the function depends on.
```matlab
% Analyzing the dependencies of the function myFunction
deps = depfun(@myFunction);
% Displaying the dependencies
disp(deps);
```
**Output:**
```
>> deps =
struct with fields:
Name: 'myFunction'
Function: 'myFunction'
Filename: 'myFunction.m'
FullPath: '/path/to/myFunction.m'
Inputs: {}
Outputs: {}
Caller: {}
Callees: {'subFunction1', 'subFunction2'}
Dependent: {'/path/to/subFunction1.m', '/path/to/subFunction2.m'}
```
**Parameter Explanation:**
* `Name`: Function name
* `Function`: Function handle
* `Filename`: Function file path
* `FullPath`: Function file absolute path
* `Inputs`: List of function input parameters
* `Outputs`: List of function output parameters
* `Caller`: List
0
0
相关推荐
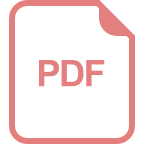
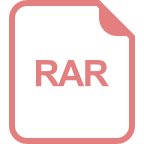
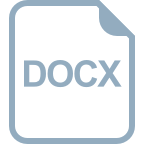
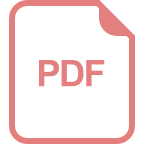
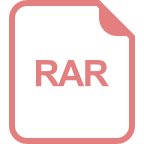
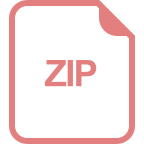
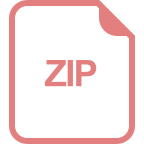