Unveiling MATLAB Path Management Black Technology: Avoid Common Mistakes, Enhance Code Readability
发布时间: 2024-09-14 13:38:01 阅读量: 9 订阅数: 17 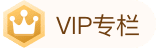
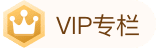
# Demystifying MATLAB Path Management: Avoid Common Mistakes and Enhance Code Readability
## 1. Overview of MATLAB Path Management
Effective organization and management of files and folders in the MATLAB workspace hinge on proper path management. A path is a list of locations where MATLAB looks for files and functions. Managing paths ensures that MATLAB can locate the necessary code and data, which in turn improves code readability, maintainability, and reproducibility. This chapter will outline the importance of MATLAB path management and introduce basic concepts and best practices.
## 2. Tips for MATLAB Path Management
### 2.1 Setting and Managing Paths
#### 2.1.1 Adding and Removing Paths
MATLAB offers several methods for adding and removing paths, with the most straightforward being the `addpath` and `rmpath` functions.
```matlab
% Adding a path
addpath('/path/to/directory');
% Removing a path
rmpath('/path/to/directory');
```
**Argument Explanation:**
* `/path/to/directory`: The path to be added or removed.
**Code Logic:**
* The `addpath` function adds the specified path to MATLAB's search path.
* The `rmpath` function removes the specified path from MATLAB's search path.
#### 2.1.2 Path Priority and Search Order
MATLAB's search path is an ordered list, with MATLAB searching for functions and data files based on priority from the list. The top of the path list has the highest priority.
```matlab
% Getting the search path
path = pathdef;
% Setting the search path
pathdef('/path/to/new/pathdef.m');
```
**Argument Explanation:**
* `/path/to/new/pathdef.m`: The MATLAB script file containing the new search path definition.
**Code Logic:**
* The `pathdef` function returns the current search path.
* The `pathdef` function can be used to set a new search path.
### 2.2 Path Dependencies and Code Readability
#### 2.2.1 Impact of Path Dependencies
Path dependency refers to the execution of code relying on its current path settings, which can lead to issues with code portability and maintainability.
**Example:**
```matlab
% Current path: /path/to/project
load('data.mat'); % Loading the data file
% Changing the current path
cd('/new/path');
% Attempting to load the data file (fails)
load('data.mat');
```
**Impact:**
* The code relies on the `data.mat` file in the current path.
* After changing paths, the code will be unable to load the data file.
#### 2.2.2 Path Management Practices for Improving Code Readability
To enhance code readability and maintainability, consider the following path management practices:
***Using Relative Paths:** Relative paths are relative to the current path, which aids in code portability.
***Avoiding Hard-Coded Paths:** Hard-coded paths bind code to specific locations, limiting code reusability.
***Using Path Variables:** Store commonly used paths in path variables to simplify code readability and maintenance.
***Using Path Management Toolboxes:** MATLAB provides path management toolboxes with functions and tools for managing paths.
## 3. MATLAB Path Management in Practice
**3.1 Avoiding Common Path Errors**
Common mistakes in MATLAB path management can affect code execution and maintainability.
**3.1.1 Traps with Absolute and Relative Paths**
MATLAB supports both absolute and relative paths. Absolute paths start from the root directory, while relative paths start from the current directory. When using relative paths, be aware of the following traps:
* Relative paths depend on the current directory. If the current directory changes, the code may not run correctly.
* Relative paths can result in poor code portability since the current directory may differ on different computers.
**3.1.2 File Name and Path Matching Issues**
When MATLAB searches paths, it matches file names and paths. If the file name and path are the same, MATLAB may prioritize loading the file from the path over the file in the current directory. For example:
```matlab
% Current directory
pwd
/home/user/project
% Adding a path
addpath('/home/user/project/lib')
% Creating a file with the same name
cd lib
touch myfile.m
cd ..
% Running the file
myfile
```
In this case, MATLAB will run `lib/myfile.m` instead of `project/myfile.m`. To avoid this issue, use absolute paths or different file names.
**3.2 Path Management Toolboxes**
MATLAB provides several toolboxes to assist with path management:
**3.2.1 Using pathtool**
`pathtool` is a graphical interface that conveniently allows users to view and manage MATLAB paths. It enables adding, removing, and reordering paths, as well as setting path priorities.
**3.2.2 Applying addpath and rmpath Functions**
The `addpath` and `rmpath` functions dynamically add and remove paths. They accept path strings or directory structures as arguments. For example:
```matlab
% Adding a path
addpath('/home/user/project/lib')
% Removing a path
rmpath('/home/user/project/lib')
```
These functions provide programming control over path management and can be used in scripts and functions.
## 4.1 Best Practices for Path Management
### 4.1.1 Path Standardization and Consistency
In large projects, maintaining standardized and consistent paths is crucial for ensuring code readability, maintainability, and collaboration. Here are some best practices:
- **Using Absolute Paths:** Absolute paths start from the root directory, clearly specifying the full path to the file. This eliminates the issues associated with relative paths.
- **Keeping Paths Short:** When using relative paths, try to keep them short. Avoid nested subdirectories or lengthy path names.
- **Using Path Aliases:** For frequently used paths, create path aliases. This simplifies code and enhances readability.
- **Using Path Delimiters:** Always use the correct path delimiters depending on the operating system (Windows: \, Unix/Linux: /).
### 4.1.2 Path Version Control and Collaboration
In a team collaboration environment, managing path version control is vital to ensure all team members use the same paths and to prevent conflicts. Here are some best practices:
- **Storing Paths in Version Control Systems:** Store path information in version control systems (like Git) to track changes and collaborate.
- **Using Path Management Tools:** Utilize path management tools (like pathtool) to manage paths and ensure consistency.
- **Establishing Path Conventions:** Establish clear path conventions and document them in team documentation.
## 4.2 Automating Path Management
### 4.2.1 Path Management for Scripts and Functions
Scripts and functions can automate path management tasks. Here are some examples:
- **Creating Path Setup Scripts:** Write a script to set up project paths and add them to the MATLAB search path.
- **Using addpath and rmpath Functions:** Use addpath and rmpath functions in scripts or functions to dynamically add and remove paths.
- **Using Path Caching:** Use path caching to store frequently used paths, thereby improving performance.
### 4.2.2 Path Management in CI/CD Tools
Integrating path management in Continuous Integration/Continuous Delivery (CI/CD) tools can ensure path consistency throughout the development process. Here are some examples:
- **Setting Paths in CI/CD Pipelines:** Include steps in CI/CD pipelines to set project paths.
- **Using Containerization:** Use containerization technologies to isolate paths and ensure consistency across different environments.
- **Leveraging Cloud Services:** Use cloud service path management features (like AWS CodeBuild).
# 5.1 Path Caching and Optimization
### 5.1.1 Mechanism and Impact of Path Caching
MATLAB caches path information in memory when loading functions or data. This caching mechanism significantly improves the efficiency of subsequent loads, as MATLAB does not need to search the paths again.
However, path caching can also present some issues:
- **Cache Inconsistency:** When paths change, the cache may not update promptly, leading to loading errors.
- **Memory Consumption:** Caching path information consumes memory, especially when many paths are involved.
- **Loading Delays:** In some cases, cached path information may not match the actual paths, causing loading delays.
### 5.1.2 Strategies for Optimizing Path Caching
To optimize path caching, consider the following strategies:
- **Regularly Clearing the Cache:** Use the `clear path` or `rehash` commands to regularly clear the path cache, ensuring it matches the actual paths.
- **Using Relative Paths:** Relative paths are not cached, thus avoiding cache inconsistency issues.
- **Reducing the Number of Paths:** Combining paths or using path toolboxes can reduce the number of paths, easing the burden on the cache.
- **Using Path Virtualization:** Path virtualization technology can separate actual paths from cached paths, avoiding cache inconsistency and memory consumption problems.
0
0
相关推荐
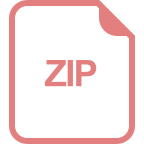
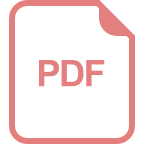
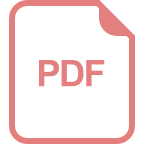
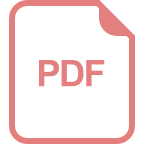
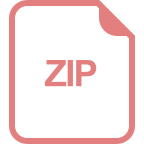