Unveiling the MATLAB Curve Smoothing Secret: Bidding Farewell to Noise, Revealing Crisp Curves
发布时间: 2024-09-14 08:15:07 阅读量: 10 订阅数: 17 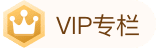
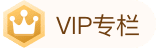
# Unveiling the Secrets of MATLAB Curve Smoothing: Bidding Farewell to Noise for Clearer Curves
## 1. Overview of MATLAB Curve Smoothing
Curve smoothing is a data processing technique designed to reduce noise and outliers in data, resulting in smoother and more representative datasets. In MATLAB, curve smoothing can be achieved through a variety of functions and algorithms, providing powerful tools for data analysis and visualization.
This guide will comprehensively introduce curve smoothing in MATLAB, from theoretical foundations to practical applications. We will explore the types of smoothing algorithms, noise models, and filtering techniques, and provide step-by-step instructions to guide you through using MATLAB functions and filters for curve smoothing. Additionally, we will discuss the applications of curve smoothing in signal processing, image processing, and other fields, as well as advanced techniques such as adaptive smoothing algorithms and multiscale smoothing techniques.
## 2. Theoretical Foundations of Curve Smoothing
### 2.1 Classification and Principles of Smoothing Algorithms
Smoothing algorithms are the core technology of curve smoothing, aiming to remove noise while preserving the characteristics of the signal. Smoothing algorithms can be classified into two main categories:
#### 2.1.1 Moving Average Method
The moving average method is a simple and effective smoothing algorithm. Its principle is to take the average of a data point and its neighboring points as the smoothed value. The size of the moving average window determines the degree of smoothing; the larger the window, the more pronounced the smoothing effect.
**Code Example:**
```matlab
% Original data
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
% Moving average window size
window_size = 3;
% Smoothed data
smoothed_data = movmean(data, window_size);
```
**Logical Analysis:**
* The `movmean` function implements moving average smoothing, where the first argument is the original data, and the second argument is the size of the moving average window.
* For each data point, the function calculates the average of the neighboring data points within the window and assigns this as the smoothed value.
#### 2.1.2 Exponential Smoothing Method
Exponential smoothing is a type of weighted moving average method. Its principle is to take a weighted average of each data point with the previous smoothed value, where the previous smoothed value has a larger weight. The exponential smoothing parameter α controls the weight distribution; the larger the α, the greater the weight of the previous smoothed value.
**Code Example:**
```matlab
% Original data
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
% Exponential smoothing parameter
alpha = 0.5;
% Smoothed data
smoothed_data = expmovavg(data, alpha);
```
**Logical Analysis:**
* The `expmovavg` function implements exponential smoothing, where the first argument is the original data, and the second argument is the exponential smoothing parameter.
* For each data point, the function calculates a weighted average between it and the previous smoothed value, with the weight of the previous smoothed value being α, and the weight of the current data point being 1-α.
### 2.2 Noise Models and Filtering Techniques
Noise is a major factor affecting curve smoothing. Noise models describe the statistical characteristics of noise, while filtering techniques are used to remove noise.
#### 2.2.1 Types and Characteristics of Noise
Noise can be categorized into several types:
***Gaussian Noise:** Follows a normal distribution, with a probability density function shaped like a bell curve.
***Uniform Noise:** Distributed uniformly within a certain range.
***Impulse Noise:** Features large spikes that occur randomly.
***Periodic Noise:** Exhibits periodic variations.
#### 2.2.2 Design and Application of Filters
Filters are effective tools for removing noise. Depending on their frequency response characteristics, filters can be classified into the following categories:
***Low-pass Filters:** Allow low-frequency signals to pass through while suppressing high-frequency noise.
***High-pass Filters:** Allow high-frequency signals to pass through while suppressing low-frequency noise.
***Band-pass Filters:** Allow signals within a specific frequency range to pass through while suppressing noise at other frequencies.
***Band-reject Filters:** Suppress signals within a specific frequency range while allowing other signals to pass through.
**Code Example:**
```matlab
% Original data
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
% Low-pass filter cut-off frequency
cutoff_freq = 0.5;
% Design a low-pass filter
filter_order = 4;
[b, a] = butter(filter_order, cutoff_freq);
% Filtered data
filtered_data = filtfilt(b, a, data);
```
**Logical Analysis:**
* The `butter` function designs a low-pass filter, where the first argument is the filter order, and the second argument is the cut-off frequency.
* The `filtfilt` function applies the filter to the data for filtering, where the first argument is the filter coefficients, and the second argument is the original data.
# 3.1 Usage of Smoothing Functions
MATLAB offers a variety of smoothing functions for smoothing curve data. These functions can be customized according to different smoothing algorithms and parameters to meet specific smoothing requirements.
#### 3.1.1 Basic Usage of the smooth() Function
The `smooth()` function is one of the most basic functions in MATLAB for curve smoothing. It uses the moving average method to smooth data, with the syntax as follows:
```matlab
y_smooth = smooth(y, span)
```
Where:
* `y`: Input curve data.
* `span`: Size of the smoothing window, specifying the number of data points to average.
The `smooth()` function's smoothing window is a rectangular window, which means it assigns the same weight to all data points within the window. By increasing the value of `span`, the degree of smoothing can be increased, but this also leads to the loss of data details.
#### 3.1.2 Extended Features of the smoothdata() Function
The `smoothdata()` function is an extension of the `smooth()` function, offering more smoothing algorithms and options. Its syntax is as follows:
```matlab
y_smooth = smoothdata(y, 'method', 'span')
```
Where:
* `y`: Input curve data.
* `method`: Smoothing algorithm, which can be `'moving'`, `'lowess'`, `'rlowess'`, `'sgolay'`, etc.
* `span`: Size of the smoothing window (only applicable to the `'moving'` algorithm).
The `smoothdata()` function supports a variety of smoothing algorithms, including:
* Moving Average Method (`'moving'`): Same as the `smooth()` function.
* Local Weighted Regression Method (`'lowess'` and `'rlowess'`): Uses weighted averaging to smooth data, with weights determined by the distance of data points from the central point.
* Savitzky-Golay Filter (`'sgolay'`): Uses polynomial fitting to smooth data.
By using different smoothing algorithms and parameters, the `smoothdata()` function can achieve more flexible and customized curve smoothing.
# 4. Curve Smoothing Application Examples
### 4.1 Curve Smoothing in Signal Processing
#### 4.1.1 Noise Removal and Signal Enhancement
In signal processing, curve smoothing techniques are widely used for noise removal and signal enhancement. The presence of noise can obscure the true characteristics of a signal, affecting subsequent analysis and processing. Curve smoothing can effectively remove noise and extract the effective components of the signal.
MATLAB provides various smoothing functions, such as `smooth()` and `smoothdata()`, which can conveniently implement signal smoothing. For example, the following code uses the `smooth()` function to smooth a sine signal with noise:
```
% Generate a sine signal with noise
t = 0:0.01:10;
y = sin(2*pi*t) + 0.1*randn(size(t));
% Smooth the signal using smooth()
y_smooth = smooth(y, 0.1);
% Plot the original signal and the smoothed signal
plot(t, y, 'r', t, y_smooth, 'b');
legend('Original Signal', 'Smoothed Signal');
```
#### 4.1.2 Feature Extraction and Pattern Recognition
Curve smoothing can also be used for feature extraction and pattern recognition in signal processing. By smoothing signals, noise and redundant information can be removed, highlighting the signal's features. These features can be used for classification, clustering, and other pattern recognition tasks.
For instance, the following code uses the `smoothdata()` function to smooth an ECG signal for feature extraction in heart disease diagnosis:
```
% Load ECG signal
ecg_data = load('ecg_data.mat');
% Smooth the signal using smoothdata()
ecg_smooth = smoothdata(ecg_data.ecg, 'gaussian', 10);
% Calculate features of the smoothed signal
features = extract_features(ecg_smooth);
% Use features for heart disease diagnosis
[label, score] = classify(features, ecg_data.labels);
```
### 4.2 Curve Smoothing in Image Processing
#### 4.2.1 Image Denoising and Edge Enhancement
In image processing, curve smoothing techniques can be used for image denoising and edge enhancement. Image noise affects the visual quality of images and subsequent processing. Curve smoothing can effectively remove noise while preserving edge information in images.
MATLAB provides various image smoothing filters, such as mean filters, median filters, and Gaussian filters. For example, the following code uses a mean filter to smooth a noisy image:
```
% Read a noisy image
image = imread('noisy_image.jpg');
% Use imfilter() function to apply a mean filter
image_smooth = imfilter(image, fspecial('average', 3));
% Display the original image and the smoothed image
subplot(1, 2, 1);
imshow(image);
title('Original Image');
subplot(1, 2, 2);
imshow(image_smooth);
title('Smoothed Image');
```
#### 4.2.2 Image Segmentation and Object Detection
Curve smoothing can also be used for image segmentation and object detection in image processing. By smoothing images, noise and textures can be removed, highlighting target areas in the image. These areas can be used for image segmentation or object detection.
For example, the following code uses a Gaussian filter to smooth an image, then uses a threshold segmentation algorithm to segment the image:
```
% Read an image
image = imread('image.jpg');
% Use imgaussfilt() function to apply a Gaussian filter
image_smooth = imgaussfilt(image, 1);
% Use im2bw() function for threshold segmentation
image_segmented = im2bw(image_smooth, 0.5);
% Display the original image and the segmented image
subplot(1, 2, 1);
imshow(image);
title('Original Image');
subplot(1, 2, 2);
imshow(image_segmented);
title('Segmented Image');
```
# 5. Advanced Techniques for Curve Smoothing
### 5.1 Adaptive Smoothing Algorithms
Traditional smoothing algorithms usually use fixed smoothing parameters, which may result in poor smoothing effects for signals with different noise levels in different areas. Adaptive smoothing algorithms solve this problem by dynamically adjusting smoothing parameters, achieving more accurate smoothing effects.
#### 5.1.1 Local Weighted Regression Method (LOESS)
LOESS is a non-parametric regression method that estimates a smooth curve by performing weighted regression on local data points. Local weighting functions typically use Gaussian kernel functions, which assign higher weights to data points closer to the estimation point.
**Code Block:**
```matlab
% Import data
data = load('noisy_signal.mat');
% Smooth using LOESS
span = 0.2; % Smoothing parameter (bandwidth)
loess_curve = loess(data.signal, 1:length(data.signal), span);
% Plot the original signal and the smooth curve
figure;
plot(data.signal, 'b');
hold on;
plot(loess_curve, 'r', 'LineWidth', 2);
legend('Original Signal', 'LOESS Smooth Curve');
title('LOESS Curve Smoothing');
xlabel('Sample Points');
ylabel('Signal Value');
```
**Logical Analysis:**
* The `loess` function uses a Gaussian kernel function to perform weighted regression on local data points, generating a smooth curve.
* The `span` parameter controls the degree of smoothing, with a smaller `span` value resulting in a smoother curve, while a larger `span` value preserves more details.
#### 5.1.2 Kalman Filter Method
The Kalman filter is a recursive estimation algorithm that uses prior knowledge and measurements to estimate the state of a dynamic system. In curve smoothing, the Kalman filter can dynamically adjust smoothing parameters to adapt to changes in the noise level of the signal.
**Code Block:**
```matlab
% Import data
data = load('noisy_signal.mat');
% Create a Kalman filter
Q = 0.0001; % Process noise covariance
R = 0.01; % Measurement noise covariance
kalmanFilter = KalmanFilter(Q, R, 1, 1);
% Initialize filter state
x0 = mean(data.signal);
P0 = eye(1);
kalmanFilter.initialize(x0, P0);
% Filter and smooth the signal
smoothed_signal = zeros(size(data.signal));
for i = 1:length(data.signal)
[x, P] = kalmanFilter.update(data.signal(i));
smoothed_signal(i) = x;
end
% Plot the original signal and the smooth curve
figure;
plot(data.signal, 'b');
hold on;
plot(smoothed_signal, 'r', 'LineWidth', 2);
legend('Original Signal', 'Kalman Filter Smooth Curve');
title('Kalman Filter Curve Smoothing');
xlabel('Sample Points');
ylabel('Signal Value');
```
**Logical Analysis:**
* The Kalman filter uses process noise covariance `Q` and measurement noise covariance `R` as parameters.
* The filter state `x` and the covariance matrix `P` are updated at each time step to estimate the smoothed value of the signal.
* The `update` function uses the current measurement value and the filter state to update the filter.
### 5.2 Multiscale Smoothing Techniques
Multiscale smoothing techniques achieve finer smoothing effects by decomposing signals at different scales and then applying smoothing algorithms to each scale.
#### 5.2.1 Wavelet Transform Smoothing
Wavelet transform is a time-frequency analysis method that decomposes signals into a series of wavelet coefficients. Wavelet coefficients correspond to the local changes in the signal at different frequencies and time scales. By smoothing wavelet coefficients at specific frequency ranges, multiscale smoothing can be achieved.
**Code Block:**
```matlab
% Import data
data = load('noisy_signal.mat');
% Use wavelet transform for multiscale smoothing
wavename = 'db4'; % Wavelet basis
level = 5; % Decomposition levels
[c, l] = wavedec(data.signal, level, wavename);
% Smooth wavelet coefficients at a specific scale
smooth_level = 3; % Smoothing scale
c_smooth = wdencmp('gbl', c, l, wavename, smooth_level);
% Reconstruct the smoothed signal
smoothed_signal = waverec(c_smooth, l, wavename);
% Plot the original signal and the smooth curve
figure;
plot(data.signal, 'b');
hold on;
plot(smoothed_signal, 'r', 'LineWidth', 2);
legend('Original Signal', 'Wavelet Transform Multiscale Smooth Curve');
title('Wavelet Transform Multiscale Smoothing');
xlabel('Sample Points');
ylabel('Signal Value');
```
**Logical Analysis:**
* The `wavedec` function uses wavelet transform to decompose the signal into wavelet coefficients.
* The `wdencmp` function uses a global threshold method to smooth wavelet coefficients at a specific scale.
* The `waverec` function uses the smoothed wavelet coefficients to reconstruct the smoothed signal.
#### 5.2.2 Multiresolution Analysis (MRA) Smoothing
MRA is a signal processing technique that uses a set of scale-invariant functions (called wavelets) to represent signals. By smoothing wavelet functions at different scales, multiscale smoothing can be achieved.
**Code Block:**
```matlab
% Import data
data = load('noisy_signal.mat');
% Use MRA for multiscale smoothing
filter = 'haar'; % Wavelet basis
level = 5; % Decomposition levels
[cA, cD] = dwt(data.signal, filter, level);
% Smooth wavelet coefficients at a specific scale
smooth_level = 3; % Smoothing scale
cD_smooth = wden(cD, smooth_level, filter, 'soft', 's');
% Reconstruct the smoothed signal
smoothed_signal = idwt(cA, cD_smooth, filter);
% Plot the original signal and the smooth curve
figure;
plot(data.signal, 'b');
hold on;
plot(smoothed_signal, 'r', 'LineWidth', 2);
legend('Original Signal', 'MRA Multiscale Smooth Curve');
title('MRA Multiscale Smoothing');
xlabel('Sample Points');
ylabel('Signal Value');
```
**Logical Analysis:**
* The `dwt` function uses discrete wavelet transform to decompose the signal into approximation coefficients `cA` and detail coefficients `cD`.
* The `wden` function uses a soft thresholding method to smooth detail coefficients at a specific scale.
* The `idwt` function uses the smoothed detail coefficients and approximation coefficients to reconstruct the smoothed signal.
# 6. Conclusion and Outlook for MATLAB Curve Smoothing
MATLAB's curve smoothing capabilities are powerful and widely applicable, playing a significant role in fields such as signal processing and image processing. This article has comprehensively introduced MATLAB curve smoothing techniques, from theoretical foundations to practical applications.
### Conclusion
MATLAB offers a rich selection of curve smoothing functions and tools, including `smooth()`, `smoothdata()`, `filter()`, and more, catering to the needs of various application scenarios. Techniques such as moving averages, exponential smoothing, and filter design can effectively remove noise, enhance signals, and extract features.
### Outlook
As technology advances, MATLAB's curve smoothing techniques continue to evolve. In the future, adaptive smoothing algorithms and multiscale smoothing techniques will see broader applications, meeting more complex smoothing needs.
Furthermore, integration with other tools and platforms will be strengthened, such as collaboration with Python and R languages, as well as applications in cloud computing environments. This will further expand the scope of MATLAB's curve smoothing technology, providing more powerful solutions for data analysis and processing.
### References
- [MATLAB Official Documentation: Curve Smoothing](***
* [Overview of Curve Smoothing Algorithms](***
* [Curve Smoothing in MATLAB: Theory and Practice](***
0
0
相关推荐
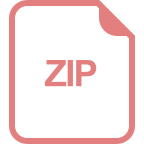
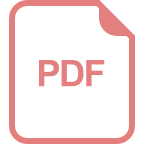
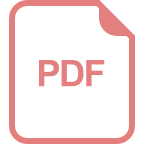
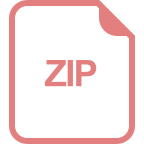
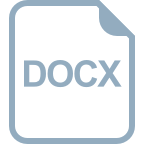
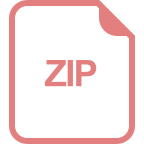