MATLAB Curve Smoothing: Eliminating Jitters for a Smooth Curve
发布时间: 2024-09-14 08:31:21 阅读量: 30 订阅数: 31 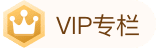
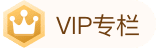
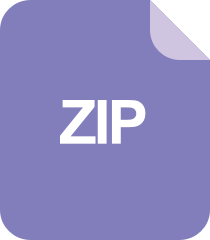
Fast Anisotropic Curvature Preserving Smoothing:Fast Anisotropic Curvature Preserving Smoothing for High Quality Image Denoising-matlab开发
# 1. Overview of Curve Smoothing
Curve smoothing is a data processing technique used to remove noise and outliers from data, revealing the underlying trends and patterns. It is widely used in signal processing, image processing, data analysis, and more.
Curve smoothing algorithms achieve this by applying weighted averages or fitting to the original data. Weighted average algorithms, such as moving averages, are simple and easy to use but may introduce lag effects. Savitzky-Golay filters are polynomial fitting-based algorithms that effectively smooth data while preserving critical features.
# 2. Theoretical Foundations
### 2.1 Curve Smoothing Algorithms
Curve smoothing algorithms aim to enhance the readability and interpretability of data by reducing noise and retaining signal characteristics. Here are three commonly used curve smoothing algorithms:
#### 2.1.1 Moving Average Method
The moving average method is a simple yet effective smoothing algorithm that smooths curves by calculating the average of neighboring data points. Its formula is as follows:
```matlab
y_smoothed = (1/n) * sum(y(i-n/2:i+n/2))
```
Where:
* `y` is the original data
* `y_smoothed` is the smoothed data
* `n` is the size of the moving window
**Parameter Description:**
* `n`: The size of the moving window. A larger window will result in a smoother curve but may lose details.
**Code Logic:**
The moving average method uses a sliding window to traverse the data, calculating the average of the data points within each window. This average replaces the data point at the center of the window, thus smoothing the curve.
#### 2.1.2 Savitzky-Golay Filter
The Savitzky-Golay filter is a smoothing algorithm based on polynomial fitting. It fits a polynomial to neighboring data points and then calculates the derivative of the polynomial at the data points to smooth the curve. Its formula is as follows:
```matlab
y_smoothed = sgolayfilt(y, order, framelen)
```
Where:
* `y` is the original data
* `y_smoothed` is the smoothed data
* `order` is the order of the polynomial
* `framelen` is the size of the filter window
**Parameter Description:**
* `order`: The order of the polynomial. A higher order will result in a smoother curve but may introduce artifacts.
* `framelen`: The size of the filter window. A larger window will result in a smoother curve but may lose details.
**Code Logic:**
The Savitzky-Golay filter traverses the data with a sliding window, fitting a polynomial within each window. It then calculates the derivative of the polynomial at the data points and uses the derivative to smooth the curve.
#### 2.1.3 Local Weighted Regression
Local weighted regression is a non-parametric smoothing algorithm that smooths curves by performing a weighted average of neighboring data points. Its formula is as follows:
```matlab
y_smoothed = lowess(y, span)
```
Where:
* `y` is the original data
* `y_smoothed` is the smoothed data
* `span` is the size of the weighted window
**Parameter Description:**
* `span`: The size of the weighted window. A larger window will result in a smoother curve but may lose details.
**Code Logic:**
Local weighted regression traverses the data with a sliding window, performing a weighted average of the data points within each window. The weights are determined by the distance of the data points from the center of the window, with closer points having greater weight.
# 3. MATLAB Implementation
### 3.1 Built-In Functions
MATLAB provides various built-in functions for curve smoothing, among which the most commonly used are `smooth` and `sgolayfilt`.
#### 3.1.1 smooth
The `smooth` function uses the moving average method to smooth data. Its syntax is:
```
y = smooth(x, span)
```
Where:
* `x` is the data sequence to be smoothed.
* `span` specifies the size of the moving window, and its value must be an odd number.
**Code Block:**
```matlab
% Generate original data
x = randn(100, 1);
% Smooth data using a moving average with span = 5
y = smooth(x, 5);
% Plot the original and smoothed data
plot(x, 'b', 'LineWidth', 1.5);
hold on;
plot(y, 'r', 'LineWidth', 1.5);
legend('Original Data', 'Smoothed Data');
```
**Logical Analysis:**
* `randn(100, 1)` generates a 100x1 sequence of normally distributed random data.
* `smooth(x, 5)` smooths `x` using the moving average method with a window size of 5.
* The `plot` function plots the original and smoothed data.
#### 3.1.2 sgolayfilt
The `sgolayfilt` function uses the Savitzky-Golay filter to smooth data. Its syntax is:
```
y = sgolayfilt(x, order, framelen)
```
Where:
* `x` is the data sequence to be smoothed.
* `order` specifies the order of the filter, and its value must be an odd number.
* `framelen` specifies the size of the filter window, and its value must be an odd number greater than `order`.
0
0
相关推荐







