MATLAB Curve Fitting Algorithm Comparison: Exploring Strengths and Weaknesses, Choosing the Best Algorithm
发布时间: 2024-09-14 08:36:33 阅读量: 22 订阅数: 20 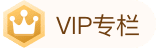
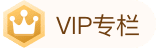
# 1. Overview of Curve Fitting
Curve fitting is a mathematical technique used to find one or more curves that approximate the trend or pattern of a given set of data points. It is widely applied in science, engineering, and data analysis fields, aiding us in understanding the inherent laws of data, and making predictions and decisions.
The purpose of curve fitting algorithms is to find a curve that minimizes the error between the curve and data points. The error measurement standards are usually indicators such as Root Mean Square Error (RMSE) or the Coefficient of Determination (R²). Different curve fitting algorithms can be employed depending on the type of data and fitting requirements, including linear regression, nonlinear regression, and interpolation algorithms.
# 2. Curve Fitting Algorithm Theory
### 2.1 Linear Regression
Linear regression is a statistical modeling technique used to fit linear relationships. It assumes that data points lie on a straight line and determines the line's parameters by minimizing the distance from data points to the line.
#### 2.1.1 Least Squares Method
The least squares method is a commonly used method in linear regression. It finds the best-fit straight line by minimizing the sum of squared errors. The sum of squared errors is defined as the sum of the squares of the distances from the data points to the line.
```python
import numpy as np
# Data points
x = np.array([1, 2, 3, 4, 5])
y = np.array([2, 4, 6, 8, 10])
# Least squares fitting
from sklearn.linear_model import LinearRegression
model = LinearRegression()
model.fit(x.reshape(-1, 1), y)
# Parameters of the fitting line
slope = model.coef_[0]
intercept = model.intercept_
# Fitting line equation
y_pred = slope * x + intercept
```
**Logical Analysis:**
* The `LinearRegression()` class is used to create a linear regression model.
* The `fit()` method fits the model and calculates the parameters.
* The `coef_` attribute contains the slope, and the `intercept_` attribute contains the intercept.
* The `y_pred` array contains the predicted y-values using the fitting line equation.
#### 2.1.2 Ridge Regression
Ridge regression is a regularized linear regression technique used to solve overfitting problems. It penalizes the size of model parameters by adding a penalty term to the objective function.
```python
from sklearn.linear_model import Ridge
# Ridge regression fitting
model = Ridge(alpha=0.1)
model.fit(x.reshape(-1, 1), y)
# Parameters of the fitting line
slope = model.coef_[0]
intercept = model.intercept_
```
**Logical Analysis:**
* The `Ridge()` class is used to create a ridge regression model.
* The `alpha` parameter controls the strength of the penalty term.
* The `coef_` and `intercept_` attributes contain the parameters of the fitting line.
### 2.2 Nonlinear Regression
Nonlinear regression is used to fit nonlinear relationships in data. It fits data points using nonlinear functions, such as polynomials or exponential functions.
#### 2.2.1 Polynomial Regression
Polynomial regression uses polynomial functions to fit data points. The order of the polynomial function determines the complexity of the fitting curve.
```python
from sklearn.preprocessing import PolynomialFeatures
from sklearn.linear_model import LinearRegression
# Polynomial feature transformation
poly_features = PolynomialFeatures(degree=2)
x_poly = poly_features.fit_transform(x.reshape(-1, 1))
# Linear regression fitting
model = LinearRegression()
model.fit(x_poly, y)
# Parameters of the fitting curve
coeffs = model.coef_
```
**Logical Analysis:**
* The `PolynomialFeatures()` class is used to transform the data into polynomial features.
* The `degree` parameter specifies the order of the polynomial.
* The `fit_transform()` method transforms the data and fits it into polynomial features.
* The `coef_` attribute contains the coefficients of the polynomial function.
#### 2.2.2 Exponential Regression
Exponential regression uses exponential functions to fit data points. The exponential function has the following form:
```
y = a * e^(bx)
```
```python
from scipy.optimize import curve_fit
# Exponential function
def exp_func(x, a, b):
return a * np.exp(b * x)
# Exponential regression fitting
popt, pcov = curve_fit(exp_func, x, y)
# Parameters of the fitting curve
a = popt[0]
b = popt[1]
```
**Logical Analysis:**
* The `curve_fit()` function is used to fit the curve to the data.
* The `exp_func` defines the exponential function.
* The `popt` array contains the fitting parameters `a` and `b`.
* The `pcov` array contains the covariance matrix.
### 2.3 Interpolation Algorithms
Interpolation algorithms are used to estimate values between data points. They work by constructing a smooth curve that passes through the data points.
#### 2.3.1 Linear Interpolation
Linear interpolation uses straight lines to connect two data points, assuming that the data between them is linear.
```python
from numpy import interp
# Linear interpolation
y_interp = interp(x_new, x, y)
```
**Logical Analysis:**
* The `interp()` function performs linear interpolation.
* `x_new` are the new data points to be interpolated.
* `y_interp` are the inter
0
0
相关推荐
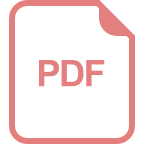
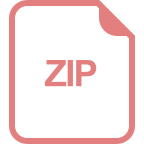
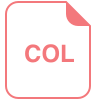
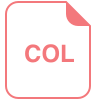
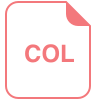
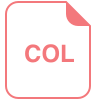
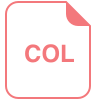
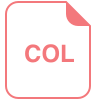