Exploring MATLAB Gaussian Fitting Optimization Algorithm: Enhancing Fitting Efficiency, Optimizing Computational Performance
发布时间: 2024-09-14 19:33:13 阅读量: 21 订阅数: 29 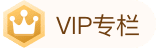
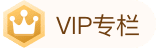
# Exploring MATLAB's Gaussian Fitting Optimization Algorithm: Enhancing Fitting Efficiency and Computational Performance
## 1. Overview of MATLAB Gaussian Fitting
Gaussian fitting is a statistical modeling technique used to fit data points to a Gaussian distribution, also known as a normal distribution. In MATLAB, the Gaussian fitting functionality is powerful and user-friendly, making it a common tool in scientific computation and data analysis.
MATLAB provides a variety of Gaussian fitting algorithms, including basic and optimized algorithms. The basic algorithms estimate Gaussian distribution parameters using the least squares method, while the optimized algorithms employ iterative methods to minimize fitting errors. By adjusting algorithm parameters, fitting accuracy can be optimized, and the robustness of the results can be improved.
## 2. Theoretical Basis of Gaussian Fitting
### 2.1 Introduction to Gaussian Distribution
The Gaussian distribution, also known as the normal distribution, is a continuous probability distribution characterized by its probability density function:
```
f(x) = (1 / (σ * √(2π))) * e^(-(x - μ)² / (2σ²))
```
Where:
* μ: the mean of the distribution
* σ: the standard deviation of the distribution
The shape of the Gaussian distribution is a bell curve, featuring:
* Symmetry around the mean μ
* The curve widens and the peak lowers as σ increases
* The curve reaches its maximum at μ
* Inflection points occur at μ ± σ
### 2.2 The Principle of Least Squares
The least squares method is an optimization technique used to fit data points to a mathematical model. In Gaussian fitting, the principle of least squares aims to find a set of parameters that minimizes the squared error between the fitted curve and the data points.
For n data points (x₁, y₁), (x₂, y₂), ..., (xₙ, yₙ), the fitted curve is:
```
y = f(x; a₁, a₂, ..., aₙ)
```
Where a₁, a₂, ..., aₙ are the fitting parameters.
The principle of least squares finds the optimal parameters by minimizing the following objective function:
```
E = Σᵢ=₁ⁿ (yᵢ - f(xᵢ; a₁, a₂, ..., aₙ))²
```
By solving the partial derivatives of the objective function for each parameter and setting them to zero, a set of linear equations can be obtained:
```
[A] * [a] = [b]
```
Where:
* [A] is an n × n matrix with elements Aᵢⱼ = Σᵢ=₁ⁿ xᵢ^j * f(xᵢ; a₁, a₂, ..., aₙ)
* [a] is an n × 1 parameter vector with elements a₁, a₂, ..., aₙ
* [b] is an n × 1 vector with elements bᵢ = Σᵢ=₁ⁿ yᵢ * xᵢ^j
Solving this system of equations yields the best fitting parameters.
# 3.1 Basic Gaussian Fitting Function
MATLAB provides the `fit` function for executing Gaussian fitting. This function takes data and the fitting model as input and returns fitting parameters and statistical information.
```matlab
% Define data
x = [1, 2, 3, 4, 5];
y = [2.1, 3.2, 4.5, 5.8, 6.9];
% Create a Gaussian model
model = fittype('a * exp(-((x - b) / c)^2)');
% Perform fitting
fitresult = fit(x', y', model);
```
**Code Logic Analy
0
0
相关推荐








