1编写前序递归算法; 2.编写中序递归算法; 3.编写后序递归算法;C++数据结构
时间: 2024-10-19 22:14:22 浏览: 27
在C++中,树是一种常见的数据结构,其中递归算法用于遍历节点。以下是三种常见递归遍历二叉树的算法:
1. **前序遍历(Preorder Traversal)递归算法**:
```cpp
void preorderRecursion(TreeNode* node) {
if (node != nullptr) {
// 先访问根节点
cout << node->data << " ";
// 然后遍历左子树
preorderRecursion(node->left);
// 最后遍历右子树
preorderRecursion(node->right);
}
}
```
在这个过程中,先打印当前节点的数据,再对左子树进行递归操作,最后处理右子树。
2. **中序遍历(Inorder Traversal)递归算法**:
```cpp
void inorderRecursion(TreeNode* node) {
if (node != nullptr) {
// 遍历左子树
inorderRecursion(node->left);
// 访问根节点
cout << node->data << " ";
// 遍历右子树
inorderRecursion(node->right);
}
}
```
这里首先递归左子树,然后访问根节点,最后递归右子树,适合按照元素值排序输出。
3. **后序遍历(Postorder Traversal)递归算法**:
```cpp
void postorderRecursion(TreeNode* node) {
if (node != nullptr) {
// 遍历左子树
postorderRecursion(node->left);
// 遍历右子树
postorderRecursion(node->right);
// 最后访问根节点
cout << node->data << " ";
}
}
```
后序遍历会先处理子节点,最后才访问根节点,常用于计算表达式或打印元素后再删除它们。
阅读全文
相关推荐
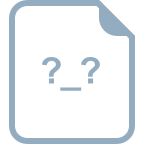
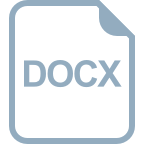
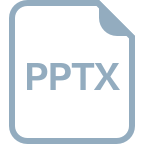
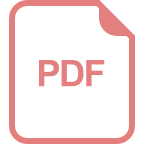
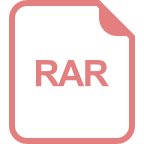
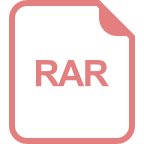
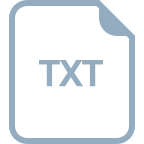
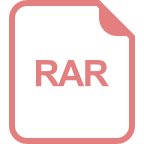
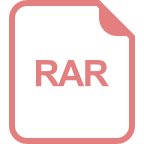
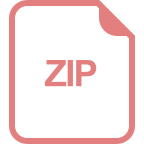
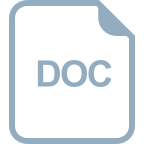
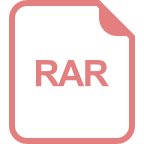
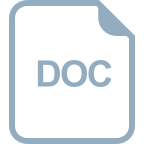
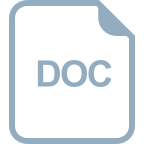