Java 用GUI和线程实现一个打字小游戏,程序每隔一段时间随机给出一个汉字(汉字字符在unicode中范围为19968-40869),玩家在输入框内输入正确即得分
时间: 2024-03-24 21:36:44 浏览: 56
好的,我可以为您提供一份基本的代码框架,您可以根据您的需求进行修改和完善:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Random;
public class TypingGame extends JFrame implements ActionListener {
private JLabel scoreLabel, chineseLabel;
private JTextField inputField;
private JButton startButton;
private int score = 0;
private char currentChinese = '\u0000';
private static final int INTERVAL = 15; // 每个汉字出现的时间间隔,单位为秒
private Thread timerThread;
public TypingGame() {
this.setTitle("打字小游戏");
this.setSize(300, 200);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setLayout(new GridLayout(4, 1));
scoreLabel = new JLabel("得分: " + score);
this.add(scoreLabel);
chineseLabel = new JLabel("请在下方输入框内输入汉字");
chineseLabel.setHorizontalAlignment(JLabel.CENTER);
this.add(chineseLabel);
inputField = new JTextField();
inputField.addActionListener(this);
inputField.setHorizontalAlignment(JTextField.CENTER);
inputField.setEnabled(false);
this.add(inputField);
startButton = new JButton("开始游戏");
startButton.addActionListener(this);
this.add(startButton);
this.setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() == startButton) {
startButton.setEnabled(false);
inputField.setEnabled(true);
inputField.requestFocus();
currentChinese = getRandomChinese();
chineseLabel.setText(String.valueOf(currentChinese));
timerThread = new Thread(new TimerRunnable());
timerThread.start();
} else if (e.getSource() == inputField) {
String inputText = inputField.getText();
if (inputText.length() > 0) {
char inputChar = inputText.charAt(0);
if (inputChar == currentChinese) {
score++;
scoreLabel.setText("得分: " + score);
currentChinese = getRandomChinese();
chineseLabel.setText(String.valueOf(currentChinese));
inputField.setText("");
}
}
}
}
private char getRandomChinese() {
Random random = new Random();
return (char) (random.nextInt(20902) + 19968);
}
private class TimerRunnable implements Runnable {
@Override
public void run() {
int timeLeft = INTERVAL;
while (timeLeft >= 0) {
try {
Thread.sleep(1000);
timeLeft--;
if (timeLeft == 0) {
gameover();
break;
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
private void gameover() {
startButton.setEnabled(true);
inputField.setEnabled(false);
inputField.setText("");
scoreLabel.setText("得分: " + score);
chineseLabel.setText("游戏结束");
}
public static void main(String[] args) {
new TypingGame();
}
}
```
这段代码使用了Java的Swing GUI库和线程机制,实现了一个简单的打字小游戏。在游戏开始后,程序会随机选择一个汉字,显示在游戏窗口中央的标签中,同时启动一个计时器线程,计时器线程会在一定时间后结束游戏。玩家需要在下方的输入框中输入正确的汉字,输入正确后得分加1,程序会再次随机选择一个汉字进行下一轮游戏。
您可以根据您的需求对代码进行修改和完善,比如增加更多的汉字,改变汉字出现的时间间隔,设计更丰富的界面等。
阅读全文
相关推荐



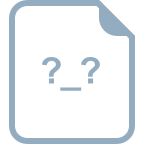
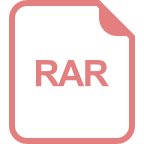
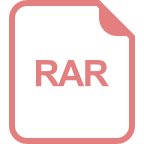
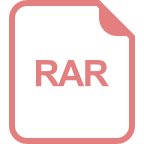
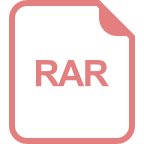
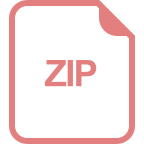
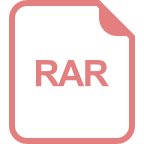
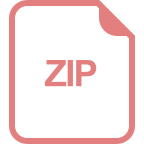
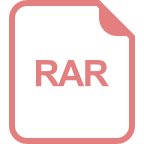
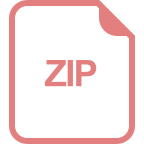
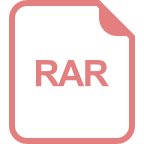
