python数组移除指定元素
时间: 2024-02-03 18:07:08 浏览: 80
要移除Python数组中的指定元素,你可以使用列表推导式或者使用`remove()`方法。这里有两种方法可以实现:
方法一:使用列表推导式
```python
arr = [1, 2, 3, 4, 5]
target = 3
new_arr = [x for x in arr if x != target]
print(new_arr)
```
输出:
```
[1, 2, 4, 5]
```
方法二:使用`remove()`方法
```python
arr = [1, 2, 3, 4, 5]
target = 3
while target in arr:
arr.remove(target)
print(arr)
```
输出:
```
[1, 2, 4, 5]
```
相关问题
python 数组移除元素
在 Python 中,可以使用 `remove()` 方法从列表中移除指定的元素。例如,如果要从一个列表中移除元素 `x`,可以使用以下语句:
```
my_list.remove(x)
```
这将从 `my_list` 列表中移除元素 `x`。
如果要移除列表中的所有指定元素,可以使用一个循环来遍历列表并移除所有匹配的元素。例如:
```
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
to_remove = [2, 4, 6, 8]
for x in to_remove:
while x in my_list:
my_list.remove(x)
print(my_list)
```
在上面的例子中,我们首先定义了一个列表 `my_list`,其中包含数字 1 到 10。然后我们定义了一个数组 `to_remove`,其中包含要从 `my_list` 中移除的数字。我们使用一个循环遍历 `to_remove` 中的每个数字,并在 `my_list` 中使用一个 while 循环移除所有匹配的元素。
最后,我们打印出 `my_list`,其中包含了从中移除了 `to_remove` 中指定的所有数字的结果。
python移除数组中的元素
在Python中,你可以使用几种方法来移除列表(数组)中的特定元素:
1. **`remove()`** 方法:这个方法会从列表中删除第一个匹配给定值的元素。如果元素不存在,会抛出 `ValueError`。例如:
```python
list = [1, 2, 3, 4, 5]
list.remove(3) # 删除第一个3,列表变为 [1, 2, 4, 5]
```
2. **`del` 关键字**:可以直接通过索引或切片操作删除指定位置的元素。例如:
```python
list = [1, 2, 3, 4, 5]
del list # 删除索引为1的元素,列表变为 [1, 3, 4, 5]
```
3. **列表推导式** 或者 `filter()` 函数:如果你想保留原列表不变,可以创建一个新的列表,过滤掉不需要的元素。例如:
```python
list = [1, 2, 3, 4, 5]
new_list = [x for x in list if x != 3] # 创建新列表,不包含3,新列表为 [1, 2, 4, 5]
```
4. **pop() 和 popitem()** 方法:`pop(index)` 可以按索引删除并返回元素,`popitem()` 删除并返回最后一个元素及其下标。
```python
list = [1, 2, 3, 4, 5]
list.pop(2) # 删除索引为2的元素,列表变为 [1, 2, 4, 5]
```
阅读全文
相关推荐
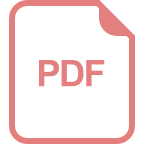
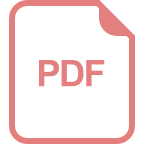
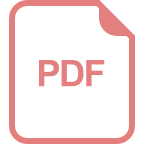

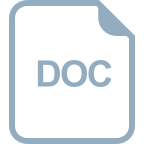
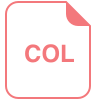






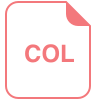



