将数值1-10存入链表中,将其逆序输出
时间: 2024-09-20 19:12:46 浏览: 43
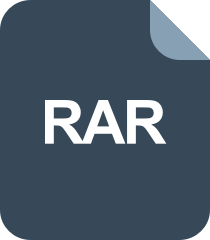
运用链表实现大数阶乘
将数字1到10存储在一个链表中并逆序输出,首先需要创建一个节点结构,每个节点包含一个数字和指向下一个节点的指针。然后可以按照以下步骤操作:
1. **初始化链表**:创建一个单向链表,并将第一个节点设为头结点,初始值设为1。
```python
class Node:
def __init__(self, value):
self.value = value
self.next = None
head = Node(1)
```
2. **添加数字到链表**:遍历从2到10的数字,依次创建新节点并插入到链表的头部。
```python
for i in range(2, 11):
new_node = Node(i)
new_node.next = head
head = new_node
```
3. **逆序链表**:创建一个新的头结点`new_head`,开始从原链表尾部遍历并将节点逐个链接到新的头结点上。
```python
new_head = None
current = head
while current:
next_temp = current.next
current.next = new_head
new_head = current
current = next_temp
```
4. **输出逆序链表**:最后,打印新的头结点`new_head`所代表的逆序链表即可。
```python
current = new_head
while current:
print(current.value, end=" ")
current = current.next
```
阅读全文
相关推荐
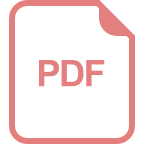
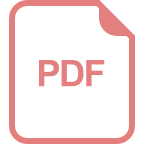



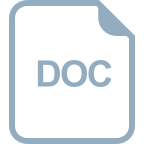
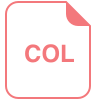

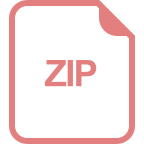
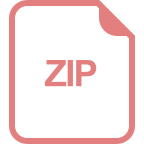