java 的webservice调用的例子
时间: 2023-09-14 11:01:17 浏览: 172
WebService是一种用于实现分布式应用程序之间通信的技术,它基于标准的SOAP协议,采用HTTP协议作为传输协议,通过XML格式进行数据交换。在Java中,使用Java的WebService框架可以轻松地创建和调用Web服务。
下面是一个Java中使用WebService调用的例子:
1. 首先,在Java中创建一个基于WebService的项目。
2. 定义一个接口,用于描述Web服务的方法。
```java
package com.example.webservice;
import javax.jws.WebService;
@WebService
public interface HelloWorld {
String sayHello(String name);
}
```
3. 实现接口,编写具体的方法实现。
```java
package com.example.webservice;
import javax.jws.WebService;
@WebService(endpointInterface = "com.example.webservice.HelloWorld")
public class HelloWorldImpl implements HelloWorld {
@Override
public String sayHello(String name) {
return "Hello, " + name + "!";
}
}
```
4. 配置Web服务,使用Java的WebService框架进行发布。
```java
package com.example.webservice;
import javax.xml.ws.Endpoint;
public class HelloWorldPublisher {
public static void main(String[] args) {
Endpoint.publish("http://localhost:8080/hello", new HelloWorldImpl());
System.out.println("Web service is running...");
}
}
```
5. 编写客户端代码,调用Web服务。
```java
package com.example.webservice;
import javax.xml.namespace.QName;
import javax.xml.ws.Service;
import java.net.URL;
public class HelloWorldClient {
public static void main(String[] args) throws Exception {
URL url = new URL("http://localhost:8080/hello?wsdl");
QName qname = new QName("http://webservice.example.com/", "HelloWorldImplService");
Service service = Service.create(url, qname);
HelloWorld hello = service.getPort(HelloWorld.class);
System.out.println(hello.sayHello("John"));
}
}
```
以上是一个简单的Java中使用WebService调用的例子。通过定义接口和实现类,然后发布Web服务,最后在客户端通过生成的代理类进行WebService调用。我们可以看到,Java中使用WebService调用非常简单,只需要使用相应的注解、配置和代理类即可实现远程调用。
阅读全文
相关推荐
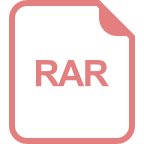
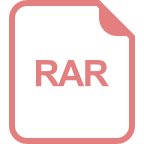
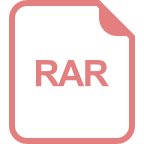
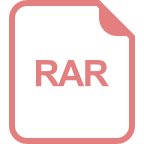
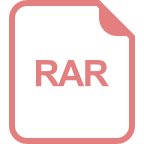
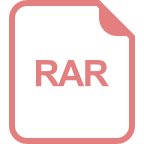
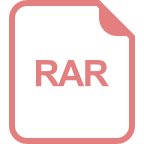
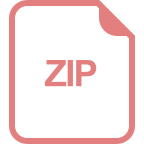
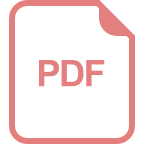
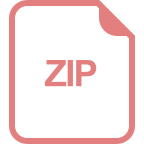
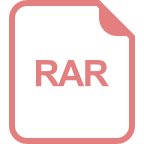
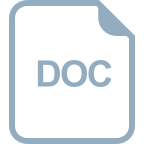
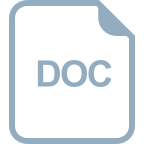
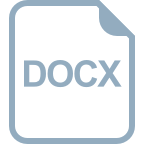
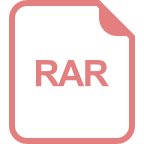
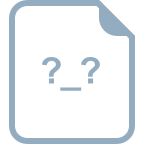
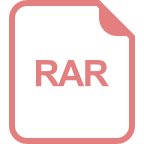