写一段程序,实现构建ResNet-Lenet-5解决Mnist数字识别,画出训练集的loss曲线和准确率取消,输出测试集的混淆矩阵、F1-score
时间: 2024-12-16 15:24:53 浏览: 20
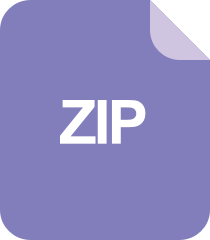
Resnet50卷积神经网络训练MNIST手写数字图像分类 Pytorch训练代码

为了实现ResNet、LeNet-5混合模型解决MNIST手写数字识别任务,并绘制训练集的损失曲线和精度图,以及计算并输出测试集的混淆矩阵和F1-score,你需要按照以下步骤编写Python代码,通常使用深度学习框架如TensorFlow或PyTorch:
首先,确保已安装所需的库:
```bash
pip install tensorflow tensorflow-addons keras matplotlib scikit-learn
```
接下来,假设我们使用Keras,这里是一个简化版本的示例:
```python
import tensorflow as tf
from tensorflow.keras.models import Sequential, Model
from tensorflow.keras.layers import Dense, Conv2D, MaxPooling2D, Flatten, Reshape, Dropout
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.applications import LeNet
from sklearn.metrics import confusion_matrix, f1_score
import matplotlib.pyplot as plt
# 加载数据
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
x_train = x_train.reshape(-1, 28, 28, 1) / 255.0
x_test = x_test.reshape(-1, 28, 28, 1) / 255.0
# 构建ResNet部分
resnet = ResNet50(weights='imagenet', include_top=False, input_shape=(28, 28, 1))
resnet_output = resnet.output
flatten = Flatten()(resnet_output)
dropout = Dropout(0.5)(flatten)
final_dense = Dense(64, activation="relu")(dropout)
# 添加LeNet-5部分
lenet_input = Input(shape=(28, 28, 1))
lenet_model = LeNet(input_shape=(28, 28, 1), classes=10)
lenet_output = lenet_model(lenet_input)
merged = tf.keras.layers.concatenate([final_dense, lenet_output])
# 输出层
output = Dense(10, activation="softmax")(merged)
# 定义模型
model = Model(inputs=[resnet.input, lenet_input], outputs=output)
model.compile(optimizer=Adam(), loss="sparse_categorical_crossentropy", metrics=["accuracy"])
# 训练模型
history = model.fit(
[x_train, x_train], y_train,
validation_data=([x_test, x_test], y_test),
epochs=10,
batch_size=128,
)
# 绘制损失曲线和准确率
plt.plot(history.history["loss"], label="Training Loss")
plt.plot(history.history["val_loss"], label="Validation Loss")
plt.title("Loss Curve")
plt.xlabel("Epochs")
plt.ylabel("Loss")
plt.legend()
plt.show()
plt.plot(history.history["accuracy"], label="Training Accuracy")
plt.plot(history.history["val_accuracy"], label="Validation Accuracy")
plt.title("Accuracy Curve")
plt.xlabel("Epochs")
plt.ylabel("Accuracy")
plt.legend()
plt.show()
# 预测
y_pred = model.predict([x_test, x_test])
y_pred_classes = np.argmax(y_pred, axis=-1)
# 混淆矩阵
conf_mat = confusion_matrix(y_test, y_pred_classes)
print("Confusion Matrix:")
print(conf_mat)
# F1-score
f1 = f1_score(y_test, y_pred_classes, average='weighted')
print(f"F1-Score: {f1}")
```
注意:此代码示例假设你已经包含了ResNet50模型,并且`ResNet50(weights='imagenet')`可能需要网络环境支持下载ImageNet预训练权重。此外,这个例子可能需要进一步调整以适应MNIST数据集的输入形状。
阅读全文
相关推荐
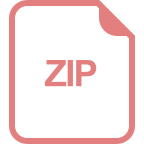
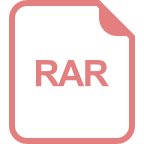

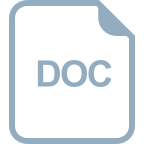













