帮我用c语言实现以下内容:1、用单链表作存储结构,定义链表结点,构造通讯录数据结构。 2、实现通讯录链表的创建、遍历、输出、清空、销毁、求表长,以及联系人信息的插人、删除、修改、查找等操作
时间: 2024-11-09 18:25:59 浏览: 23
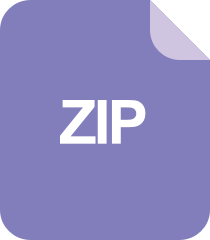
通讯录的制作(数据结构c语言 代码+报告)

首先,让我们来构建C语言中的链表节点,用于表示通讯录中的联系人信息。这包括姓名、电话号码和其他详细信息:
```c
// 定义链表节点结构体
typedef struct Node {
char name[50]; // 姓名
char Tel_1[20], Tel_2[20]; // 两个电话号码
char HomeTel[20], OfficeTel_1[20], OfficeTel_2[20]; // 其他电话和地址
char position[50]; // 职位
char post[50]; // 电邮
char mail_address[100]; // 通信地址
struct Node* next; // 指向下一个节点
} Contact;
```
接下来,我们可以实现以下功能:
1. **创建链表**:
```c
// 创建新节点并插入到链表尾部
void insertContact(Contact** head, const char* name, ...){
Contact* newNode = (Contact*)malloc(sizeof(Contact));
va_list args;
va_start(args, name);
strcpy(newNode->name, name);
// ...从args中填充其他字段
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
} else {
Contact* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
va_end(args);
}
```
2. **遍历和输出**:
```c
// 从头开始输出联系人信息
void outputContacts(Contact* head) {
OutputNode(head); // 使用已有的OutputNode函数
}
```
3. **链表操作**:
- **求表长**:
```c
int listLength(Contact* head) {
return ListLength(head); // 使用已有的ListLength函数
}
- **插入、删除、修改和查找**:
这些操作涉及到链表节点的动态管理,具体实现会依赖于链表的操作细节,例如使用迭代或递归遍历找到要修改的节点,然后更新或删除。这里仅给出基本框架:
```c
// 插入:在特定位置插入新联系人
void insertAtPosition(int pos, Contact** head, ...) {...}
// 删除:根据名称或其他标识符删除联系人
void deleteContact(Contact** head, const char* name) {...}
// 修改:找到并更新联系人的信息
void updateContact(Contact** head, const char* oldName, const char* newName, ...) {...}
// 查找:返回匹配给定条件的第一个联系人
Contact* findContact(Contact* head, const char* name) {...}
```
4. **清空和销毁链表**:
```c
void clearList(Contact** head) {
Contact* current = *head;
Contact* temp;
while (current != NULL) {
temp = current;
current = current->next;
free(temp);
}
*head = NULL;
}
```
阅读全文
相关推荐
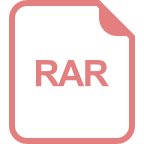
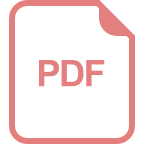

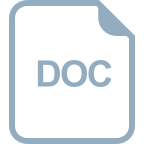

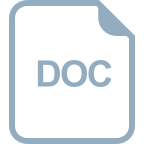
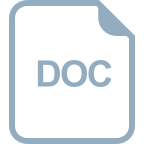

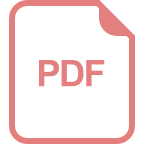
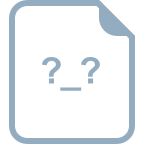
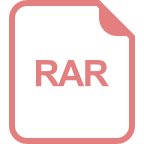
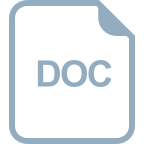
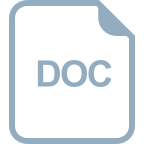
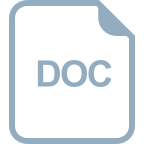
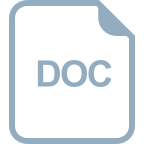
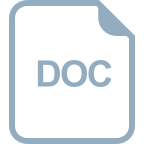

