用C语言实现数据结构单链表的删除
时间: 2024-09-18 07:10:35 浏览: 42
在C语言中,单链表是一种常用的数据结构,它由节点组成,每个节点包含数据域和指向下一个节点的指针。要从链表中删除某个元素,我们需要遍历链表并找到目标节点,然后更新前一个节点的指针使其指向目标节点的下一个节点。以下是一个简单的删除操作的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data; // 节点存储的数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 删除指定值的节点函数
void deleteNode(Node** head, int value) {
if (*head == NULL) { // 如果链表为空,直接返回
return;
}
// 遍历链表
Node* current = *head;
while (current != NULL && current->data != value) {
if (current->next == NULL) {
printf("Value %d not found in the list.\n", value);
return; // 未找到指定值,结束遍历
}
current = current->next;
}
// 找到目标节点后进行删除
if (current != NULL) {
if (current == *head) { // 如果目标节点是头节点
*head = current->next;
} else { // 否则,更新前一个节点的next指针
Node* prev = current->prev;
prev->next = current->next;
}
free(current); // 释放已删除节点的内存
}
}
// 示例:创建和删除链表中的节点
int main() {
Node* head = malloc(sizeof(Node)); // 初始化头节点
head->data = 1;
head->next = malloc(sizeof(Node));
head->next->data = 2;
head->next->next = malloc(sizeof(Node));
head->next->next->data = 3;
head->next->next->next = NULL;
printf("Before deletion:\n");
printList(head);
deleteNode(&head, 2); // 删除值为2的节点
printf("\nAfter deletion:\n");
printList(head);
return 0;
}
// 辅助函数,用于打印链表
void printList(Node* node) {
while (node != NULL) {
printf("%d -> ", node->data);
node = node->next;
}
printf("NULL\n");
}
阅读全文
相关推荐
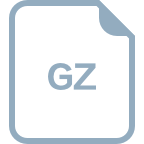
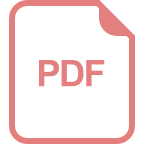
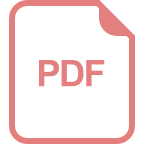
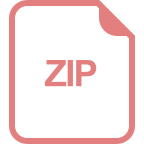
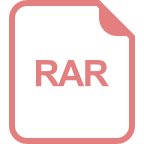
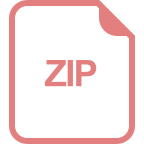
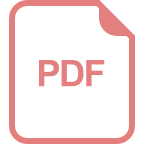
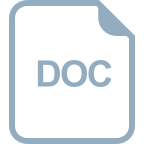
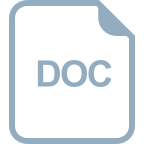
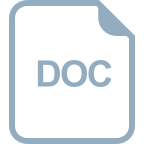
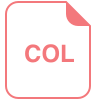

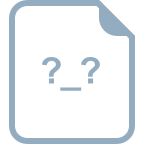
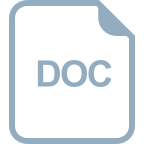



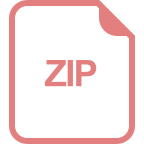
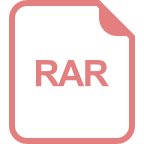