/** * 获取 list 是否为空(true) * @return list 是否为空 */ boolean isEmpty();数组方法实现代码
时间: 2024-02-26 22:50:07 浏览: 100
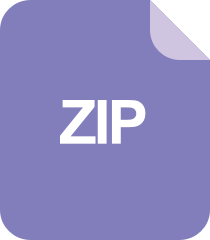
vb.net获得wifi名称及密码
Sure! Here is an example implementation of the `isEmpty()` method using an array:
```java
public class MyList {
private int[] array;
public MyList() {
array = new int[0];
}
public boolean isEmpty() {
return array.length == 0;
}
}
```
In this example, the `isEmpty()` method checks if the length of the array is 0, indicating that the list is empty. If the length is 0, it returns `true`; otherwise, it returns `false`.
阅读全文
相关推荐
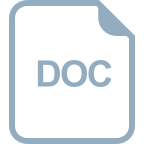
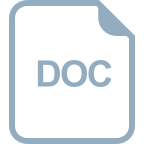















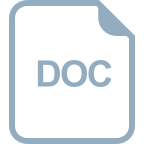