std::string::find
时间: 2024-10-24 20:12:24 浏览: 35
`std::string::find`是C++标准库中的成员函数,它用于在一个字符串对象中查找指定字符、子串或模式首次出现的位置。这个函数有几种版本:
1. `size_t find(char c) const`: 查找字符`c`第一次出现在字符串中的位置,如果找不到则返回`npos`(通常是一个特殊值,表示未找到)。
2. `size_t find(const char* str, size_t pos = 0) const`: 在给定起始位置`pos`开始查找子串`str`,如果找到就返回对应的索引,未找到则返回`npos`。
3. `size_t find(std::string_view substr, size_t pos = 0) const`: 类似于`const char*`版本,但接受`std::string_view`类型的子串作为查找目标。
4. `size_t find_first_of(const string& str, size_t pos = 0) const`: 查找第一个出现在`str`中的字符,并从位置`pos`开始查找。
5. `size_t find_last_of(const string& str, size_t pos = npos) const`: 从字符串尾部向前查找,寻找第一个出现在`str`中的字符。
需要注意的是,所有这些函数都是大小写敏感的,如果你想进行不区分大小写的搜索,你需要先对字符串进行转换或者手动处理。此外,`find`返回的是子串的第一个字符的索引,而不是整个子串的长度。
相关问题
std::string::copy, std::string::find, std::basic_stringbuf<char>::str(), std::basic_ios<char>::widen(char), std::ostream::operator<<(int)
这些是 C++ 标准库中几个不同类和成员函数的简要介绍:
1. `std::string::copy`:这个成员函数用于将源字符串的一部分复制到目标字符串指定的位置。它接受两个参数,一个是源字符串,另一个是目标字符串以及起始位置,返回的是目标字符串的新结束位置。
示例:
```cpp
std::string source = "Hello";
std::string dest("World");
dest.copy(source.begin() + 1, source.end());
```
2. `std::string::find`:查找给定子串在字符串中的位置,如果找到则返回子串的第一个字符的索引,未找到则返回 `npos`。注意它区分大小写。
示例:
```cpp
std::string str("Hello World");
size_t pos = str.find("World"); // 返回6,因为"World"从索引6开始
```
3. `std::basic_stringbuf<char>::str()`:这是 `basic_stringbuf` 类的一个方法,通常用在缓冲区(如输入/输出流缓冲)操作后获取其存储的内容作为一个可修改的`std::string`。它是一个读取操作,不会改变原始缓冲区的状态。
4. `std::basic_ios<char>::widen(char)`:此函数用于将单个字符转换为宽字符(通常是 UTF-16 或 UTF-32),以便于处理多字节字符集。它扩展了基本 I/O 流类,支持不同编码格式的文本处理。
5. `std::ostream::operator<<(int)`:这是 `std::ostream` 类的一个重载运算符,当向流(如 `cout`)中插入整数时被调用,将整数值输出到流中,便于格式化输出。
例如,打印一个整数:
```cpp
int num = 123;
std::cout << num; // 输出 "123"
```
这些都是 C++ 字符串和流操作中常用的函数或运算符,它们各自服务于不同的功能需求。
std::map<std::string,std::mapstd::string,std::string> my_map;如何只插入内层map的第一个元素
对于`std::map<std::string, std::map<std::string, std::string>> my_map;`,如果你只想插入内层map的第一个元素,可以按照以下步骤进行操作:
1. 首先,你需要检查外层map中是否已经存在指定的键。如果不存在,你需要先插入一个新的键值对。
2. 接下来,你可以通过外层map的迭代器找到指定的键,并获取对应的内层map。
3. 然后,你可以使用内层map的insert函数插入第一个元素。
下面是示例代码:
```cpp
#include <iostream>
#include <map>
#include <string>
int main() {
std::map<std::string, std::map<std::string, std::string>> my_map;
// 检查外层map中是否存在指定的键
std::string outer_key = "outer_key";
if (my_map.find(outer_key) == my_map.end()) {
// 如果不存在,插入新的键值对
my_map.insert({outer_key, std::map<std::string, std::string>()});
}
// 获取内层map并插入第一个元素
std::string inner_key = "inner_key";
std::string value = "value";
my_map[outer_key].insert({inner_key, value});
// 输出结果
for (const auto& outer_pair : my_map) {
std::cout << "Outer Key: " << outer_pair.first << std::endl;
for (const auto& inner_pair : outer_pair.second) {
std::cout << "Inner Key: " << inner_pair.first << ", Value: " << inner_pair.second << std::endl;
}
}
return 0;
}
```
这样,你就可以只插入内层map的第一个元素了。
阅读全文
相关推荐
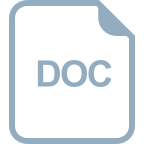
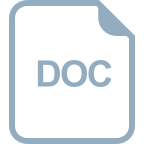
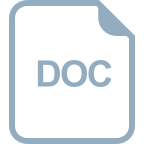













