kotlin MVI的示例代码
时间: 2024-08-09 22:01:01 浏览: 35
Kotlin MVIs是一种简化MVI(MultiView-Intent-Response)模式实现的库,它可以用于构建响应式UI组件。下面是一段使用Kotlin MVIs基本框架的简单示例代码:
首先,我们需要导入必要的依赖项:
```kotlin
dependencies {
implementation "com.github.hierynomus:kotlin-mvi:0.4.0"
}
```
然后,我们定义一个简单的应用状态(State)和一些动作(Action):
```kotlin
import com.github.hierynomus.mvi.State
import com.github.hierynomus.mvi.actions.Action
data class AppState(
val counter: Int = 0,
)
sealed interface AppAction : Action<AppState> {
object IncrementCounter : AppAction
}
class CounterViewState : State<AppState>() {
override fun render(state: AppState): String =
"${state.counter} count"
}
```
接下来,我们将定义一个处理AppAction的Handler:
```kotlin
class CounterHandler : com.github.hierynomus.mvi.Handler<AppState, AppAction>() {
override fun handle(action: AppAction, state: AppState) = when (action) {
is AppAction.IncrementCounter -> AppState(counter = state.counter + 1)
else -> throw IllegalArgumentException("Unknown action")
}
}
```
最后,我们将创建视图并启动MVI应用:
```kotlin
fun main() {
val initialState = AppState()
val view = CounterViewState()
val handler = CounterHandler()
// 观察应用状态的变化并更新视图
handler.observeChanges(initialState, { newState ->
view.onNewState(newState)
})
// 发送动作并观察结果
handler.send(AppAction.IncrementCounter)
println(view.render()) // 输出 '1 count'
handler.send(AppAction.IncrementCounter)
println(view.render()) // 输出 '2 count'
}
```
这个例子展示了如何使用Kotlin MVIs构建一个简单的计数应用。当发送增加计数值的动作时,会触发对应的处理器函数,更新应用的状态,并通知视图进行渲染展示新的内容。这种方式非常适合构建响应式UI,能够更好地分离关注点,让代码结构更加清晰明了。
-
相关推荐
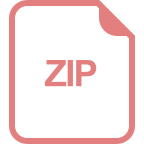
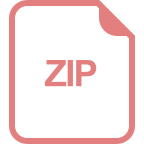
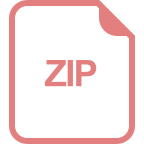














