java编程序: 实现一个复数类Complex, 成员函数包括加、减、乘、除、打印、返回实部、虚部 和 toString()方法等, 其中toString()返回 a+bi形式的字符串。 成员函数:Add/Sub/Multiply/Divide不修改对象值,而是返回一个新对象。
时间: 2024-10-27 13:05:38 浏览: 58
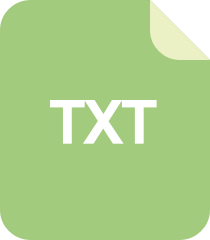
java .定义一个复数类 complex ,它的内部具有两个实例变量:realPart和imagPart,分别代表复数的实部和虚

在Java中,我们可以创建一个名为`Complex`的类来表示复数,并提供你所提到的各种成员函数。这是一个简单的例子:
```java
public class Complex {
private double real;
private double imaginary;
// 构造函数,初始化复数的实部和虚部
public Complex(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
// 获取实部和虚部
public double getReal() {
return real;
}
public double getImaginary() {
return imaginary;
}
// 加法,返回一个新的复数对象
public Complex add(Complex other) {
double newReal = this.real + other.getReal();
double newImaginary = this.imaginary + other.getImaginary();
return new Complex(newReal, newImaginary);
}
// 减法,返回一个新的复数对象
public Complex subtract(Complex other) {
double newReal = this.real - other.getReal();
double newImaginary = this.imaginary - other.getImaginary();
return new Complex(newReal, newImaginary);
}
// 乘法,返回一个新的复数对象
public Complex multiply(Complex other) {
double newReal = this.real * other.getReal() - this.imaginary * other.getImaginary();
double newImaginary = this.real * other.getImaginary() + this.imaginary * other.getReal();
return new Complex(newReal, newImaginary);
}
// 除法,返回一个新的复数对象(假设除数非零)
public Complex divide(Complex divisor) {
double denominator = divisor.getReal() * divisor.getReal() + divisor.getImaginary() * divisor.getImaginary();
double newReal = (this.real * divisor.getReal() + this.imaginary * divisor.getImaginary()) / denominator;
double newImaginary = (this.imaginary * divisor.getReal() - this.real * divisor.getImaginary()) / denominator;
return new Complex(newReal, newImaginary);
}
// 打印复数,如"a + bi"的形式
@Override
public String toString() {
if (imaginary >= 0) {
return real + " + " + imaginary + "i";
} else {
return real + " - " + (-imaginary) + "i";
}
}
}
```
在这个类中,所有的操作都不会直接修改对象的状态,而是通过返回新的`Complex`实例来体现复数运算的特性。
阅读全文
相关推荐
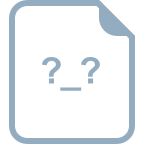
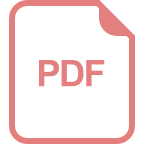















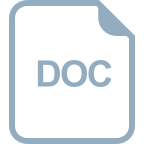