springboot调用webservice接口,数据格式为json
时间: 2023-09-09 15:08:45 浏览: 85
在Spring Boot中调用Web Service接口,并且数据格式为JSON格式,可以按照以下步骤进行操作:
1. 添加相关依赖:在pom.xml文件中添加以下依赖,以支持Web Service和JSON的相关功能。
```xml
<dependencies>
<!-- Spring Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Web Services -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web-services</artifactId>
</dependency>
<!-- Jackson JSON Processor -->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
</dependency>
</dependencies>
```
2. 创建一个Web Service客户端:使用Spring Boot提供的`WebServiceTemplate`类来创建一个Web Service客户端。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.ws.client.core.support.WebServiceGatewaySupport;
@Component
public class WebServiceClient extends WebServiceGatewaySupport {
@Autowired
public WebServiceClient() {
// 设置WebService服务的URL
setDefaultUri("http://example.com/your-webservice-url");
}
public YourResponseObject callWebService(YourRequestObject request) {
// 使用WebServiceTemplate发送请求并获取响应
YourResponseObject response = (YourResponseObject) getWebServiceTemplate()
.marshalSendAndReceive(request);
return response;
}
}
```
3. 创建请求和响应对象:根据你的Web Service接口定义,创建对应的请求和响应对象。
```java
import javax.xml.bind.annotation.XmlRootElement;
@XmlRootElement
public class YourRequestObject {
// 请求参数
private String parameter1;
private String parameter2;
// getter和setter方法
// ...
}
@XmlRootElement
public class YourResponseObject {
// 响应数据
private String result1;
private String result2;
// getter和setter方法
// ...
}
```
4. 创建Controller:创建一个控制器类,用于处理客户端的请求。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class YourController {
@Autowired
private WebServiceClient webServiceClient;
@PostMapping("/your-endpoint")
public YourResponseObject callWebService(@RequestBody YourRequestObject request) {
YourResponseObject response = webServiceClient.callWebService(request);
return response;
}
}
```
以上就是在Spring Boot中调用Web Service接口并使用JSON格式进行数据交互的基本步骤。根据你的具体情况,可能还需要根据实际需求进行一些额外的配置和处理。
相关推荐
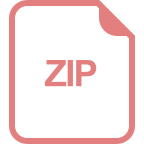
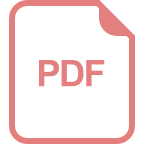














