python调用webservice调用接口传数据
时间: 2023-10-29 15:05:21 浏览: 68
使用 Python 调用 WebService 接口传输数据,可以使用 Python 标准库自带的 suds 模块。下面是一个简单的示例代码:
```python
from suds.client import Client
# 创建 WebService 客户端
client = Client('http://webservice.example.com/Service.asmx?WSDL')
# 准备要传输的数据
data = {
'param1': 'value1',
'param2': 'value2'
}
# 调用 WebService 接口
result = client.service.method_name(data)
# 处理返回结果
print(result)
```
其中,`http://webservice.example.com/Service.asmx?WSDL` 是 WebService 接口的 WSDL 地址,`method_name` 是要调用的接口方法名,`data` 是要传输的数据。调用成功后,返回的结果保存在 `result` 变量中,可以根据具体情况进行处理。
相关问题
python调用webservice接口
可以使用 Python 的第三方库如 suds、zeep 或 requests 来调用 webservice 接口。首先需要确定 webservice 接口的 WSDL 地址,然后根据不同的库使用相应的方法进行调用。例如使用 suds 库可以这样调用:
```
from suds.client import Client
wsdl_url = 'http://example.com/webservice?wsdl'
client = Client(wsdl_url)
result = client.service.some_method(param1, param2)
```
使用 requests 库可以这样调用:
```
import requests
url = 'http://example.com/webservice'
params = {'param1': 'value1', 'param2': 'value2'}
headers = {'content-type': 'application/soap+xml'}
response = requests.post(url, data=data, headers=headers)
```
使用 zeep 库可以这样调用:
```
from zeep import Client
wsdl_url = 'http://example.com/webservice?wsdl'
client = Client(wsdl_url)
result = client.service.some_method(param1, param2)
```
此外,您还可以使用 `suds-jurko` 库,它是 `suds` 库的一个扩展版本,提供了更多的功能。
python调用webservice接口授权访问
要调用WebService接口需要进行授权访问,通常有两种方式:基于用户名密码的授权和基于令牌的授权。
基于用户名密码的授权是指在调用WebService接口时,需要提供用户名和密码来进行身份验证。具体实现方式可以参考以下Python代码:
```python
import requests
from requests.auth import HTTPBasicAuth
url = 'https://webservice.example.com/api'
username = 'your_username'
password = 'your_password'
response = requests.get(url, auth=HTTPBasicAuth(username, password))
print(response.content)
```
其中,`HTTPBasicAuth`是requests库提供的基于用户名密码的身份验证方法。在这个例子中,我们使用`requests.get`方法来调用WebService接口,并传入`auth`参数来进行身份验证。
基于令牌的授权是指在调用WebService接口时,需要提供一个令牌来进行身份验证。这个令牌通常是由WebService服务端生成,并在一定时间内有效。具体实现方式可以参考以下Python代码:
```python
import requests
url = 'https://webservice.example.com/api'
token = 'your_token'
headers = {'Authorization': f'Bearer {token}'}
response = requests.get(url, headers=headers)
print(response.content)
```
其中,`Bearer`是HTTP协议中的一种身份验证机制,表示我们使用的是基于令牌的授权方式。在这个例子中,我们使用`requests.get`方法来调用WebService接口,并传入`headers`参数来携带身份验证信息。
相关推荐
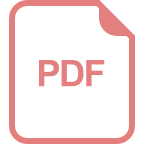
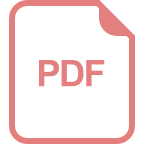












