Python绘制以姓名为x轴,y轴表示各次作业成绩的条形图
时间: 2024-09-19 10:01:34 浏览: 33
在Python中,你可以使用matplotlib库来创建这样的条形图。首先,你需要有一个包含姓名和对应成绩的数据集。下面是一个简单的例子:
```python
import matplotlib.pyplot as plt
# 假设你的数据存储在一个字典或列表中,格式如下:
data = {
'张三': [85, 90, 92],
'李四': [88, 95, 91],
'王五': [87, 93, 94]
}
# 获取最大值作为y轴范围
max_score = max([sum(sub_list) for sub_list in data.values()])
names = list(data.keys())
scores = [sum(sub_list) for sub_list in data.values()]
# 创建条形图
plt.bar(names, scores)
plt.xlabel('姓名') # x轴标签
plt.ylabel('总成绩') # y轴标签
plt.title('姓名与各次作业成绩分布') # 图表标题
plt.ylim(0, max_score + 10) # 调整y轴刻度范围
# 显示图形
plt.show()
```
这个脚本会生成一个每个姓名对应的平均成绩的条形图。如果你的实际数据结构不同,只需相应地调整数据提取部分。
相关问题
用Python绘制一个条形图,x轴表示所在部分,y轴表示平均收入,柱子的颜色表明是否离职
为了在 Python 中绘制条形图,你需要安装一个可视化库。Matplotlib 是 Python 中最常用的可视化库之一,它可以轻松地绘制各种图表。
首先,导入 matplotlib 库中的 pyplot 模块。
```
import matplotlib.pyplot as plt
```
然后,你需要准备数据。这里假设你已经有了一个包含所在部分、平均收入和是否离职的数据集。你可以使用列表或 NumPy 数组存储数据。
接下来,使用 plt.bar() 函数绘制条形图。在绘制条形图之前,你还需要调用 plt.xlabel() 和 plt.ylabel() 函数为 x 轴和 y 轴添加标签,使用 plt.title() 函数为图表添加标题。
示例代码如下所示:
```
# 准备数据
department = ['HR', 'R&D', 'Marketing', 'Sales']
salary = [50000, 80000, 45000, 90000]
left = [0, 0, 1, 1] # 0 表示未离职,1 表示已离职
# 绘制条形图
plt.bar(department, salary, color=left)
# 添加标签
plt.xlabel('Department')
plt.ylabel('Salary (USD)')
plt.title('Average Salary by Department')
# 显示图表
plt.show()
```
绘制出来的图表将会显示所在部分与平均收入之间的关系,并且柱子的颜色将表明是否离职。希望这些信息对你有帮助。
python提取电子表格数据,第一行为x轴,第一列为y轴绘制三维条形图
您可以使用Python的pandas库来读取电子表格数据,并使用Matplotlib库绘制三维条形图。以下是一个简单的示例代码:
```
import pandas as pd
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 读取电子表格数据
df = pd.read_excel('data.xlsx', index_col=0)
# 获取x轴和y轴数据
x = df.columns.values
y = df.index.values
# 将电子表格数据转换为二维数组
data = df.values
# 创建3D图形对象
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# 绘制3D条形图
for i in range(len(y)):
xs = [j for j in range(len(x))]
ys = [i] * len(x)
zs = data[i]
ax.bar(xs, zs, ys, zdir='y', alpha=0.8)
# 设置x轴和y轴标签
ax.set_xlabel('X')
ax.set_ylabel('Y')
# 显示图形
plt.show()
```
在这个示例中,我们使用pandas库的`read_excel()`方法来读取名为 `data.xlsx` 的电子表格数据。然后,我们使用`index_col`参数将第一列设置为行索引。接下来,我们获取x轴和y轴数据,将电子表格数据转换为二维数组,并创建一个3D图形对象。最后,我们使用Matplotlib的`bar()`方法绘制3D条形图,并设置x轴和y轴标签。您可以根据需要修改代码来适应您的电子表格数据。
阅读全文
相关推荐
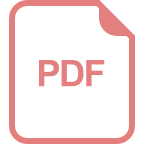
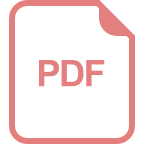
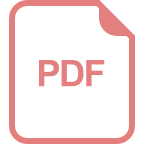













