C++的dll中参数有Mat类型的参数,C#调用C++的dll时如何编写接口函数
时间: 2024-10-26 12:03:47 浏览: 39
当你在C++ DLL中定义了一个接受`cv::Mat`作为参数的函数,而在C#中打算调用这个函数时,你需要做的是:
1. **头文件定义**:首先,在C++的DLL项目中,需要在头文件(`.h`)中声明接受`cv::Mat`的函数原型,类似这样:
```cpp
extern "C" __declspec(dllexport) void MyFunction(const cv::Mat& inputMat);
```
这里使用`__declspec(dllexport)`是为了标记该函数将在DLL中导出。
2. **实现函数**:在对应的源文件(`.cpp`)中,实现实际的函数逻辑。
3. **C#接口绑定**:在C#中,你需要使用PInvoke(Platform Invocation Services)技术来调用这个DLL。首先,生成C++的类型定义(TBB),然后创建一个C#委托或方法来匹配该函数:
```csharp
[DllImport("YourDllName.dll", CallingConvention = CallingConvention.Cdecl)]
public static extern void MyFunction([In] IntPtr matPtr, [MarshalAs(UnmanagedType.LPArray)] byte[] data, int rows, int cols, int channels);
```
注意这里假设`cv::Mat`的数据已转换为字节数组(`byte[]`),并且提供了额外的参数来描述矩阵的尺寸。
4. **传递`cv::Mat`到C++**:在C#代码中,你需要先将`cv::Mat`内容复制到`byte[]`数组,然后传给`matPtr`参数。如果可能的话,考虑使用第三方库(如SharpOpenCV或Emgu.CV)提供的包装类。
```csharp
cv::Mat inputMat = ...; // 创建或加载cv::Mat
byte[] imageData = new byte[inputMat.TotalSize];
inputMat.ToBytes(imageData);
MyFunction(new IntPtr(imageData), imageData.Length, inputMat.Rows, inputMat.Cols, inputMat.Channels());
```
阅读全文
相关推荐
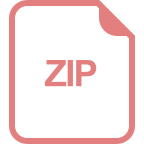
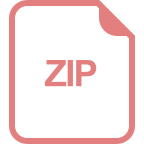
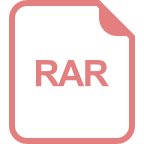
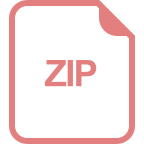
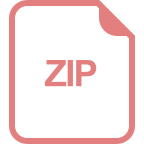
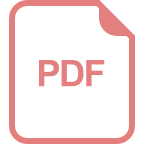
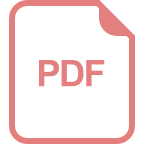
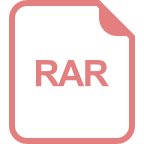
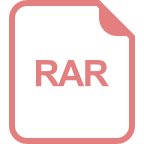
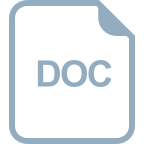








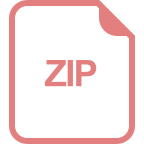