symfony增删改查
时间: 2023-09-01 08:09:41 浏览: 128
Symfony 是一个用 PHP 编写的开源 PHP Web 应用程序框架。它提供了一组工具和库,可以帮助开发者快速构建、部署和维护 Web 应用程序。
在 Symfony 中进行增删改查操作需要使用 Doctrine ORM(对象关系映射)。Doctrine ORM 是 Symfony 默认的持久化层,在数据库和对象之间建立映射关系,提供了方便的 API 来进行数据库操作。
下面是一个示例代码,演示了如何使用 Symfony 和 Doctrine ORM 进行增删改查操作:
1. 添加数据(Create):
```php
// 在控制器中引入 Doctrine
use App\Entity\YourEntity;
use Doctrine\ORM\EntityManagerInterface;
// 创建实体对象并设置属性
$yourEntity = new YourEntity();
$yourEntity->setProperty1('value1');
$yourEntity->setProperty2('value2');
// 获取 Doctrine EntityManager
$entityManager = $this->getDoctrine()->getManager();
// 将实体对象持久化到数据库
$entityManager->persist($yourEntity);
$entityManager->flush();
```
2. 查询数据(Read):
```php
// 在控制器中引入 Doctrine
use App\Repository\YourEntityRepository;
// 获取实体对象的仓库
$yourEntityRepository = $this->getDoctrine()->getRepository(YourEntity::class);
// 查询所有实体对象
$yourEntities = $yourEntityRepository->findAll();
```
3. 更新数据(Update):
```php
// 在控制器中引入 Doctrine
use App\Entity\YourEntity;
use Doctrine\ORM\EntityManagerInterface;
// 获取 Doctrine EntityManager
$entityManager = $this->getDoctrine()->getManager();
// 根据实体的主键获取实体对象
$yourEntity = $entityManager->getRepository(YourEntity::class)->find($id);
// 修改实体对象的属性
$yourEntity->setProperty1('new value1');
$yourEntity->setProperty2('new value2');
// 更新实体对象到数据库
$entityManager->flush();
```
4. 删除数据(Delete):
```php
// 在控制器中引入 Doctrine
use App\Entity\YourEntity;
use Doctrine\ORM\EntityManagerInterface;
// 获取 Doctrine EntityManager
$entityManager = $this->getDoctrine()->getManager();
// 根据实体的主键获取实体对象
$yourEntity = $entityManager->getRepository(YourEntity::class)->find($id);
// 从数据库中删除实体对象
$entityManager->remove($yourEntity);
$entityManager->flush();
```
以上是使用 Symfony 和 Doctrine ORM 进行增删改查操作的基本示例。你可以根据自己的业务需求进行相应的修改和扩展。
阅读全文
相关推荐
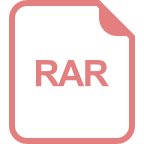
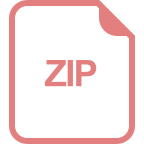
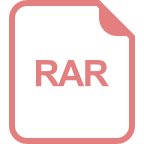
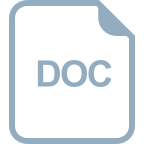
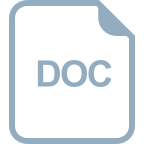
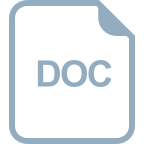
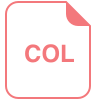
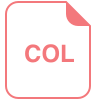
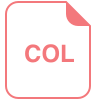

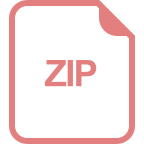
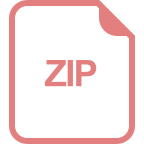
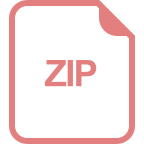
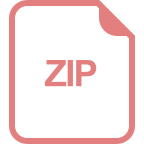
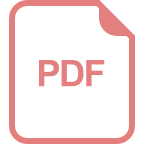
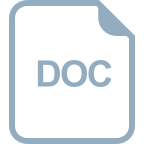
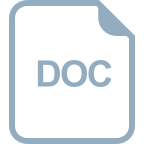