使用单链表编程实现建立一个学生单链表,并在此表中查找、插入和删除一个元素。
时间: 2024-09-24 16:24:18 浏览: 38
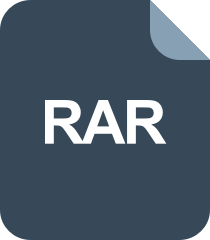
ListLink.rar_stl list_单链表_单链表 插入 删除 查找_链表 插入
在编程中,可以使用单链表结构来实现一个简单的学生信息存储系统。首先,我们需要创建节点类(Node),包含学生的属性如姓名(Name)、学号(ID)等,以及指向下一个节点的指针。然后,我们可以创建链表类(StudentList)并实现以下功能:
1. **创建节点(Insertion)**:
- `addStudent(Student student)`: 创建一个新的节点,设置学生的数据,并将其添加到链表的末尾。
2. **查找节点(Search)**:
- `findStudentById(int id)`: 遍历链表,查找具有指定学号的学生。
3. **插入节点(Insertion)**:
- `insertStudent(Student student, int position)`: 如果位置合法(0 <= position <= length),在给定位置插入新学生;否则在末尾插入。
4. **删除节点(Deletion)**:
- `deleteStudentById(int id)`: 查找具有指定学号的学生,如果找到则删除该节点。
以下是Python样例代码实现:
```python
class Node:
def __init__(self, name, id):
self.name = name
self.id = id
self.next = None
class StudentList:
def __init__(self):
self.head = None
# 插入节点
def add_student(self, student):
new_node = Node(student.name, student.id)
if not self.head:
self.head = new_node
else:
current = self.head
while current.next:
current = current.next
current.next = new_node
# 查找节点
def find_student_by_id(self, id):
current = self.head
while current and current.id != id:
current = current.next
return current is not None
# 删除节点
def delete_student_by_id(self, id):
if not self.head:
return
if self.head.id == id:
self.head = self.head.next
return
current = self.head
while current.next and current.next.id != id:
current = current.next
if current.next:
current.next = current.next.next
# 使用示例
student_list = StudentList()
student1 = Student('张三', 1001)
student2 = Student('李四', 1002)
student_list.add_student(student1)
student_list.add_student(student2)
found = student_list.find_student_by_id(1002) # 返回True
```
阅读全文
相关推荐
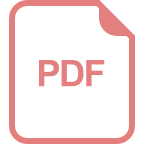
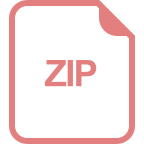

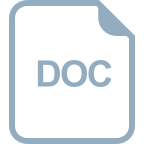
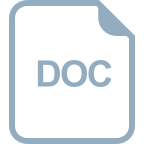
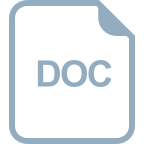









