2、已知一个列表list=[23,13,9,8,1,0,46], 先通过头插法构建单链表,然后在索引分别为0、3、5位置分别插入一个元素10,最后删除首结点,观察表的结构变化用python
时间: 2024-09-26 07:08:18 浏览: 21
在Python中,我们可以先创建一个单链表节点类`Node`,然后通过头插法将给定的列表转换成链表。接着,我们可以在指定索引处插入新的节点,最后删除首节点并打印链表结构。
首先,我们需要定义一个节点类`Node`:
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
```
然后,我们将列表转换为链表:
```python
def list_to_linked_list(lst):
if not lst:
return None
head = Node(lst[0])
current = head
for i in range(1, len(lst)):
new_node = Node(lst[i])
current.next = new_node
current = current.next
return head
# 给定列表
lst = [23, 13, 9, 8, 1, 0, 46]
head = list_to_linked_list(lst)
```
接下来,我们在索引0、3、5位置插入新元素10:
```python
def insert_at_index(head, index, value):
if index == 0:
new_node = Node(value)
new_node.next = head
return new_node
else:
current = head
for _ in range(index - 1):
current = current.next
new_node = Node(value)
new_node.next = current.next
current.next = new_node
return head
# 插入元素
head = insert_at_index(head, 0, 10) # 在头节点
head = insert_at_index(head, 3, 10) # 在索引3
head = insert_at_index(head, 5, 10) # 在索引5
# 删除首节点 (这里仅示例,实际操作需要保留指针)
head = head.next # 删除后的新头节点
```
最后,我们遍历链表并打印结构:
```python
current = head
while current is not None:
print(current.data, end=" -> ")
current = current.next
print("None") # 结束标记
```
现在你可以运行以上代码,观察链表结构的变化。
阅读全文
相关推荐
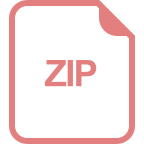
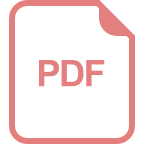
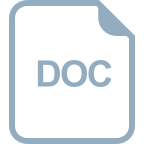















