已知列表 list1 = [3, 8, 11, 26, 47],从键盘输入一个新的数字将其插入该列表,保持列表中的元素有序排列 (要求不能使用 sort() 函数)
时间: 2024-10-16 13:11:21 浏览: 19
你可以使用 Python 的内置函数 `insert()` 和一个循环来实现这个功能,同时保证插入后的列表仍然有序。下面是一个简单的步骤说明:
1. 首先,遍历列表 `list1`,比较新输入的数字与当前元素,如果新数小于等于当前元素,则继续向后比较;如果新数大于当前元素,就将当前元素移到已排序的部分,然后插入新数。
```python
list1 = [3, 8, 11, 26, 47]
new_num = int(input("请输入新的数字:"))
i = 0 # 插入位置索引
while i < len(list1):
if new_num <= list1[i]:
break # 新数比当前元素小或相等,跳出循环
else:
list1.insert(i, list1[i]) # 移动较大元素并插入
del list1[i+1] # 删除移动过的元素,准备插入新数
i += 1
list1.insert(i, new_num) # 现在找到正确的位置,插入新数
```
现在 `list1` 就会包含按顺序排列的新数字了。
相关问题
如何使用C语言编程实现以下功能:从键盘输入创建一个动态单链表?以及如何在特定元素(已知值)前插入一个新的节点?又该如何按照给定值删除一个特定的节点?请提供相应的代码示例和步骤说明。
在C语言中,我们可以使用结构体来表示链表节点,并通过指针操作实现链表的操作。以下是创建、插入和删除动态单链表的基本步骤:
1. **创建链表**
首先,我们需要定义一个链表节点结构体:
```c
typedef struct Node {
int data; // 节点数据
struct Node* next; // 指向下一个节点的指针
} Node;
```
然后,你可以编写函数从键盘获取用户输入并创建新的节点:
```c
Node* createList() {
Node* head = NULL;
int input;
while (scanf("%d", &input) == 1) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = input;
newNode->next = head;
head = newNode;
}
return head;
}
```
这将读取用户的整数输入,直到用户不再输入为止。
2. **在特定元素前插入新节点**
我们需要遍历找到目标位置,然后插入新节点:
```c
void insertBefore(Node** head, int value, int newVal) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = newVal;
if (*head == NULL || (*head)->data == value) { // 如果链表为空或头节点就是要插入的位置
newNode->next = *head;
*head = newNode;
} else {
Node* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next != NULL) {
newNode->next = current->next;
current->next = newNode;
}
}
}
```
这个函数接受链表头指针的地址作为参数,如果链表为空或头节点就是目标值,就直接插入到头;否则,找到目标值的前一个节点并插入。
3. **删除特定节点**
使用类似的方式遍历链表找到要删除的节点,然后连接前后节点:
```c
void deleteNode(Node** head, int value) {
if (*head == NULL) return;
if ((*head)->data == value) { // 删除头节点
Node* temp = *head;
*head = (*head)->next;
free(temp);
} else {
Node* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next != NULL) {
Node* temp = current->next;
current->next = current->next->next;
free(temp);
}
}
}
```
这里,如果头节点是要删除的节点,则移除头节点并释放内存;如果不是,继续查找直到找到目标节点并删除它。
记得在完成每个操作之后检查是否成功分配了内存,并在适当的地方处理错误情况。这是链表操作的一些基本实现,更复杂的功能可以根据实际需求进行扩展。
(1)随机产生或键盘输入一组元素,建立一个带头结点的单向链表(无序)。(2)遍历单向链表。 (3)把单向链表中元素逆置(不允许申请新的结点空间)。(4)在单向链表中删除所有的偶数元素结点。 (5)编写在非递减有序链表中插入一个元素使链表元素仍有序的雨数,并利用该函数建立一个非递减有序单向链表。 (6)利用算法5建立两个非递减有序单向链表,然后合并成一个非递增链表。(7)利用算法5建立两个非递减有序单向链表,然后合并成一个非递减链表。(8)利用算法1建立的链表,实现将其分解成两个链表,其中一个全部为奇数,另一个全部为偶数(尽量利用已知的存储空间)。 (9)在主函数中设计一个简单的菜单,分别调试上述算法。
我可以回答你的第一个问题。以下是实现这些功能的代码:
```python
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = Node()
def add(self, data):
new_node = Node(data)
cur = self.head
while cur.next != None:
cur = cur.next
cur.next = new_node
def display(self):
elems = []
cur = self.head.next
while cur != None:
elems.append(cur.data)
cur = cur.next
print(elems)
def reverse(self):
prev = None
cur = self.head.next
while cur != None:
next_node = cur.next
cur.next = prev
prev = cur
cur = next_node
self.head.next = prev
def remove_even(self):
cur = self.head.next
prev = self.head
while cur != None:
if cur.data % 2 == 0:
prev.next = cur.next
cur = cur.next
else:
prev = cur
cur = cur.next
def insert_sorted(self, data):
new_node = Node(data)
cur = self.head.next
prev = self.head
while cur != None and cur.data < data:
prev = cur
cur = cur.next
new_node.next = cur
prev.next = new_node
def merge_sorted(self, other):
new_list = LinkedList()
cur1 = self.head.next
cur2 = other.head.next
while cur1 != None and cur2 != None:
if cur1.data < cur2.data:
new_list.add(cur1.data)
cur1 = cur1.next
else:
new_list.add(cur2.data)
cur2 = cur2.next
while cur1 != None:
new_list.add(cur1.data)
cur1 = cur1.next
while cur2 != None:
new_list.add(cur2.data)
cur2 = cur2.next
return new_list
def split_odd_even(self):
odd_list = LinkedList()
even_list = LinkedList()
cur = self.head.next
while cur != None:
if cur.data % 2 == 0:
even_list.add(cur.data)
else:
odd_list.add(cur.data)
cur = cur.next
return odd_list, even_list
# 测试代码
lst = LinkedList()
lst.add(3)
lst.add(2)
lst.add(4)
lst.add(1)
lst.display() # [3, 2, 4, 1]
lst.reverse()
lst.display() # [1, 4, 2, 3]
lst.remove_even()
lst.display() # [1, 3]
lst.insert_sorted(2)
lst.insert_sorted(4)
lst.insert_sorted(5)
lst.display() # [1, 2, 3, 4, 5]
lst2 = LinkedList()
lst2.add(2)
lst2.add(3)
lst2.add(6)
lst2.add(7)
lst2.add(8)
lst2.display() # [2, 3, 6, 7, 8]
lst3 = lst.merge_sorted(lst2)
lst3.display() # [1, 2, 2, 3, 3, 4, 5, 6, 7, 8]
odd_list, even_list = lst3.split_odd_even()
odd_list.display() # [1, 3, 3, 5, 7]
even_list.display() # [2, 2, 4, 6, 8]
```
你可以根据需要在主函数中调用这些方法。
阅读全文
相关推荐
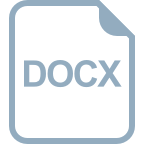
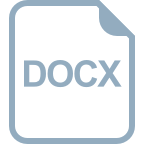
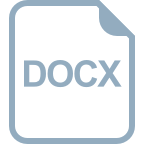
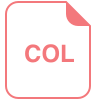
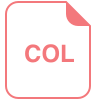
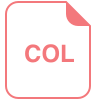
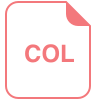
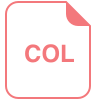




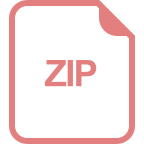
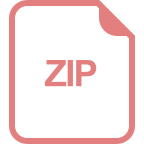
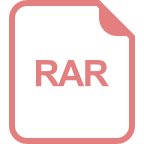
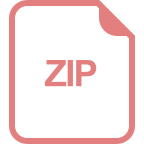
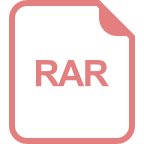