建立顺序队列并用C语言实现入队出队操作,并编写主函数来调用上述函数体
时间: 2024-11-13 07:24:29 浏览: 30
在C语言中,我们可以使用数组来实现一个简单的顺序队列。顺序队列的基本操作包括入队(enqueue)和出队(dequeue)。这里是一个基础的实现示例:
```c
#include <stdio.h>
#define MAX_SIZE 10
typedef struct {
int data[MAX_SIZE];
int front; // 队头指针
int rear; // 队尾指针
} Queue;
// 初始化队列
void init(Queue *q) {
q->front = -1;
q->rear = -1;
}
// 判断队列是否为空
int is_empty(const Queue *q) {
return q->front == -1;
}
// 判断队列是否已满
int is_full(const Queue *q) {
return (q->rear + 1) % MAX_SIZE == q->front;
}
// 入队操作
void enqueue(Queue *q, int value) {
if (is_full(q)) {
printf("Queue is full.\n");
return;
}
if (is_empty(q)) {
q->front = q->rear = 0;
} else {
q->rear = (q->rear + 1) % MAX_SIZE;
}
q->data[q->rear] = value;
printf("Enqueued %d\n", value);
}
// 出队操作
int dequeue(Queue *q) {
if (is_empty(q)) {
printf("Queue is empty.\n");
return -1;
}
int value = q->data[q->front];
if (q->front == q->rear) {
q->front = q->rear = -1;
} else {
q->front = (q->front + 1) % MAX_SIZE;
}
printf("Dequeued %d\n", value);
return value;
}
// 主函数演示队列操作
int main() {
Queue queue;
init(&queue);
enqueue(&queue, 1);
enqueue(&queue, 2);
enqueue(&queue, 3);
dequeue(&queue);
dequeue(&queue);
return 0;
}
```
这个程序首先定义了一个顺序队列结构`Queue`,包含了数据数组、队头和队尾指针。然后实现了初始化、判断空/满、入队和出队的函数。在`main`函数中,我们创建了一个队列实例并展示了基本的入队和出队操作。
阅读全文
相关推荐
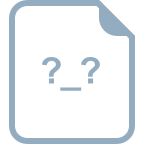
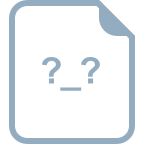
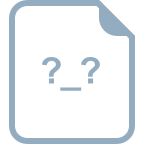
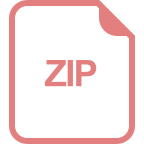
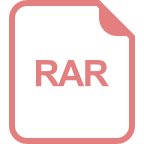
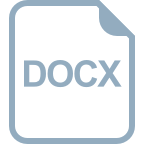
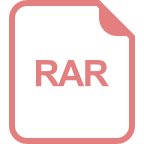
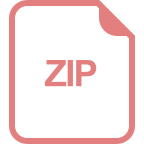
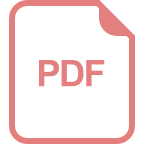
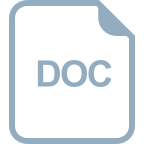
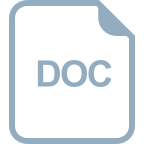
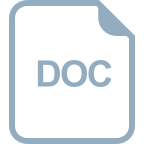
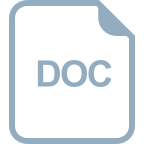