python 数组去重
时间: 2023-08-16 17:16:50 浏览: 54
要对Python数组进行去重,你可以使用set()函数或者使用列表推导式。下面是两种常见的方法:
1. 使用set()函数:
```python
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = list(set(my_list))
print(unique_list)
```
输出:
```
[1, 2, 3, 4, 5]
```
2. 使用列表推导式:
```python
my_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = []
[unique_list.append(x) for x in my_list if x not in unique_list]
print(unique_list)
```
输出:
```
[1, 2, 3, 4, 5]
```
这两种方法都可以移除重复的元素并获得一个不重复的数组。使用set()函数的方法会打乱原有的顺序,而使用列表推导式的方法可以保持原有的顺序。根据你的需求选择合适的方法。
相关问题
python 数组 去重
在Python中,数组去重可以使用多种方法实现。其中,引用中的方法使用了一个空列表s,遍历原数组arr,如果当前元素不在s中,就将其添加到s中,最后返回s即可实现去重。引用[2]中的方法使用了set数据结构,将原数组arr转换为set,然后再转换回列表即可实现去重。而引用中的方法是针对有序数组的情况,通过双指针的方式,将重复的元素覆盖掉,最后返回去重后的数组长度。根据不同的需求和数组类型,可以选择不同的方法来实现去重操作。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [数组去重大全(python)](https://blog.csdn.net/weixin_38730719/article/details/97392038)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
python数组去重
在 Python 中,可以使用 set() 函数来去除一个数组中的重复元素。具体实现方法如下:
```python
arr = [1, 2, 3, 3, 4, 4, 5]
new_arr = list(set(arr))
print(new_arr)
```
输出结果为:[1, 2, 3, 4, 5]。其中,set() 函数将一个数组转换为一个集合,集合中的元素不允许重复,最后再将集合转换为列表即可。
相关推荐
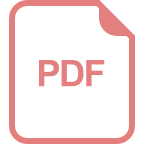












